建立带头结点的单链表,试编写算法,在第i个位置前插入一个新元素,在第j个位置删除一个元素用C语言编写,并解释代码含义
时间: 2024-09-09 15:10:15 浏览: 52
在C语言中,我们首先需要定义一个链表节点结构体`Node`,包含数据域`data`和指向下一个节点的指针`next`。然后可以编写以下函数来实现插入和删除操作:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
typedef struct Node {
int data;
struct Node* next;
} Node;
// 创建一个新的链表节点
Node* createNode(int data) {
Node* newNode = (Node*)malloc(sizeof(Node));
if (!newNode) {
printf("Memory allocation failed.\n");
return NULL;
}
newNode->data = data;
newNode->next = NULL;
return newNode;
}
// 在第i个位置前插入新元素
void insertBefore(Node** head, int i, int value) {
// 插入位置判断边界条件
if (*head == NULL || i <= 0) {
printf("Invalid insertion position.\n");
return;
}
Node* newNode = createNode(value);
if (i == 1) { // 如果是头节点
newNode->next = *head;
*head = newNode;
} else {
Node* current = *head;
for (int j = 1; j < i - 1 && current != NULL; ++j) {
current = current->next;
}
if (current != NULL) {
newNode->next = current->next;
current->next = newNode;
} else {
printf("Position out of range.\n");
}
}
}
// 删除第j个元素(1-indexed)
void deleteElement(Node** head, int j) {
if (*head == NULL || j <= 0) {
printf("Invalid deletion position.\n");
return;
}
if (j == 1) { // 如果要删除头节点
Node* temp = *head;
*head = (*head)->next;
free(temp);
} else {
Node* current = *head;
for (int i = 1; i < j - 1 && current != NULL; ++i) {
current = current->next;
}
if (current != NULL && current->next != NULL) {
Node* temp = current->next;
current->next = temp->next;
free(temp);
} else {
printf("Position out of range or list too short to delete at that index.\n");
}
}
}
// 打印链表
void printList(Node* head) {
while (head != NULL) {
printf("%d -> ", head->data);
head = head->next;
}
printf("NULL\n");
}
int main() {
Node* list = createNode(1); // 初始化一个有1的链表
list = insertBefore(&list, 2, 2); // 在第二个位置插入2
list = insertBefore(&list, 3, 3); // 在第三个位置插入3
printList(list);
deleteElement(&list, 2); // 删除第二个位置的元素(值为2)
printList(list);
return 0;
}
```
这段代码首先创建了一个简单的链表,并演示了如何在其特定位置插入和删除元素。`insertBefore`函数会在给定位置前插入新元素,而`deleteElement`函数会删除指定位置的元素。注意,索引从1开始,这意味着第一个位置对应的是`Node* next`。
阅读全文
相关推荐
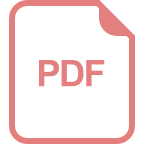





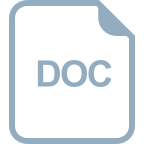









