y用c语言写一段代码,要去在单链表L中第i个位置上插入一个数据结点
时间: 2024-03-20 07:44:58 浏览: 66
好的,以下是使用C语言实现在单链表L中第i个位置上插入一个数据结点的代码:
```c
#include <stdio.h>
#include <stdlib.h>
struct Node {
int data;
struct Node* next;
};
void insert(struct Node** headRef, int data, int position) {
struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
newNode->data = data;
if (position == 1) {
newNode->next = *headRef;
*headRef = newNode;
}
else {
struct Node* temp = *headRef;
for (int i = 1; i < position - 1; i++) {
temp = temp->next;
}
newNode->next = temp->next;
temp->next = newNode;
}
}
void printList(struct Node* head) {
while (head != NULL) {
printf("%d ", head->data);
head = head->next;
}
}
int main() {
struct Node* head = NULL;
insert(&head, 10, 1);
insert(&head, 20, 2);
insert(&head, 30, 3);
printf("Linked list: ");
printList(head);
insert(&head, 15, 2);
printf("\nLinked list after inserting 15 at position 2: ");
printList(head);
return 0;
}
```
在上述代码中,我们首先定义了一个名为Node的结构体,表示链表中的每个节点。然后,我们实现了一个名为insert的函数,用于在单链表L中第i个位置上插入一个数据结点。该函数使用两个参数:headRef表示指向链表头节点的指针的指针,data表示要插入的数据,position表示插入位置。
如果插入位置position是1,则我们只需要将新节点插入到链表头部即可。否则,我们需要遍历到第position-1个节点,将新节点插入到该节点的后面。
最后,我们实现了一个名为printList的函数,用于打印链表中的所有元素。在main函数中,我们首先创建了一个空链表,然后向其中插入了三个元素。我们打印出了链表内容,然后在第二个位置上插入了一个新节点,并再次打印出链表内容。
阅读全文
相关推荐
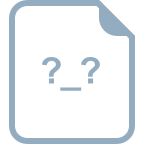
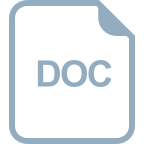
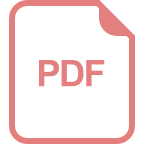
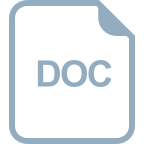
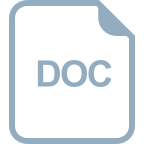
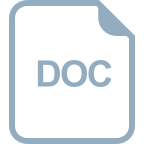
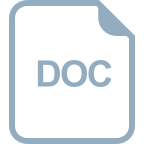
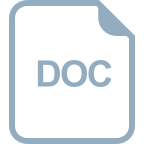

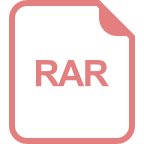
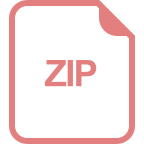
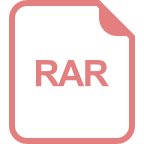