定义学生结构体struct Student类型如下: char id[10]; char Name[20]; double Score[3];门课程成绩 在主函数main()中定义struct Student类型变量stu1,从键盘输入数据赋给stu1的各成员,并修改成员数据、显示数据。增加struct Student* next成员。并以新的学生结构体struct Student类型为结点,使用malloc函数动态建立单链表。程序结束前使用free函数释放单链表所有结点。输入一个学号,在单链表上查找该学号的结点,找到,则显示该结点全部信息,找不到,则给出提示。用c语言编写
时间: 2024-03-23 19:38:22 浏览: 54
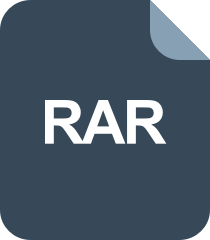
26、【C语言高级数据类型】结构体.rar
好的,我理解了你的问题。下面是代码实现,可以供你参考:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义学生结构体
struct Student {
char id[10]; // 学号
char Name[20]; // 姓名
double Score[3]; // 3门课程成绩
struct Student* next; // 指向下一个结点的指针
};
int main() {
// 定义并初始化stu1变量
struct Student stu1 = {"", "", {0}, NULL};
// 从键盘输入数据到stu1变量中
printf("请输入学生的学号:");
scanf("%s", stu1.id);
printf("请输入学生的姓名:");
scanf("%s", stu1.Name);
printf("请输入学生的3门课程成绩:");
scanf("%lf %lf %lf", &stu1.Score[0], &stu1.Score[1], &stu1.Score[2]);
// 修改stu1的成员数据
strcpy(stu1.Name, "张三");
stu1.Score[0] = 85.5;
// 显示stu1的数据
printf("学生的学号是:%s\n", stu1.id);
printf("学生的姓名是:%s\n", stu1.Name);
printf("学生的3门课程成绩是:%lf, %lf, %lf\n", stu1.Score[0], stu1.Score[1], stu1.Score[2]);
// 动态建立单链表
struct Student* head = NULL; // 链表头指针
struct Student* p = NULL; // 当前结点指针
int n = 0; // 链表长度计数器
// 输入学生信息
do {
// 创建新结点
p = (struct Student*)malloc(sizeof(struct Student));
if (p == NULL) {
printf("内存分配失败!\n");
exit(0);
}
printf("请输入第%d个学生的学号:", n+1);
scanf("%s", p->id);
printf("请输入第%d个学生的姓名:", n+1);
scanf("%s", p->Name);
printf("请输入第%d个学生的3门课程成绩:", n+1);
scanf("%lf %lf %lf", &p->Score[0], &p->Score[1], &p->Score[2]);
// 插入新结点到链表中
p->next = head;
head = p;
n++; // 链表长度+1
printf("是否继续输入学生信息?(Y/N)");
char c = getchar(); // 读取回车符
c = getchar(); // 读取用户输入的字符
if (c == 'N' || c == 'n') {
break;
}
} while (1);
// 查找给定学号的学生信息
char target_id[10];
printf("请输入要查找的学生学号:");
scanf("%s", target_id);
p = head;
while (p != NULL) {
if (strcmp(p->id, target_id) == 0) { // 找到了目标学生结点
printf("学生的学号是:%s\n", p->id);
printf("学生的姓名是:%s\n", p->Name);
printf("学生的3门课程成绩是:%lf, %lf, %lf\n", p->Score[0], p->Score[1], p->Score[2]);
break;
}
p = p->next;
}
if (p == NULL) {
printf("没有找到学号为%s的学生!\n", target_id);
}
// 遍历单链表并释放内存
p = head;
while (p != NULL) {
struct Student* temp = p;
p = p->next;
free(temp); // 释放当前结点的内存
}
return 0;
}
```
这段代码实现了以下功能:
1. 定义了一个学生结构体,包含学号、姓名、3门课程成绩、指向下一个结点的指针。
2. 从键盘输入数据到一个stu1变量中,并修改成员数据、显示数据。
3. 使用malloc函数动态建立单链表,并从用户输入中添加学生信息。
4. 根据用户输入的学号,在单链表上查找该学号的结点,找到则显示该结点全部信息,找不到则给出提示。
5. 遍历单链表并释放内存,确保程序结束前释放所有结点的内存。
阅读全文
相关推荐
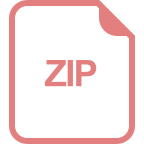
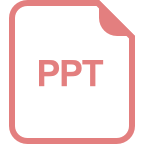



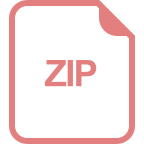
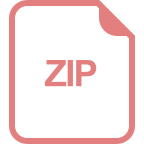
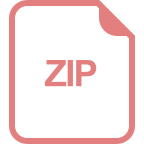
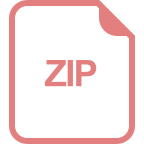
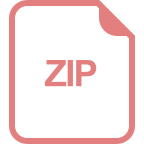
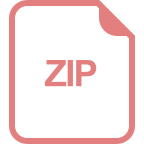
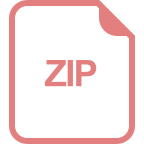
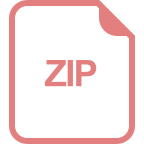
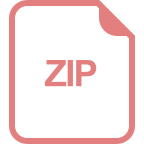
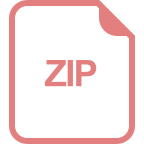
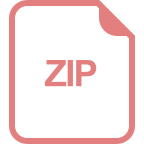
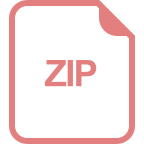