使用链表等数据结构进行优化:定义学生结构体struct Student类型如下: char id[10]; //学号 char Name[20];//姓名 double Score[3];//3门课程成绩 定义并初始化struct Student数组stus[10],显示stus数组数据,输入学生学号,查找该生的数据;删除符合某个条件的所有学生数据;建立struct Student类型的变量并赋值,将该变量插入到stus数组的末尾,依据3门课程的总成绩,对stus数组进行降序排序,如总成绩相同,则按照Score[0]降序排序,并显示。用c语言编写
时间: 2024-02-29 13:55:25 浏览: 24
以下是使用链表进行优化的C语言代码:
```c
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
// 学生结构体
struct Student {
char id[10]; // 学号
char name[20]; // 姓名
double score[3]; // 3门课程成绩
struct Student *next; // 指向下一个学生节点的指针
};
// 初始化学生链表
struct Student *init() {
struct Student *head = NULL;
struct Student *tail = NULL;
for (int i = 0; i < 10; i++) {
struct Student *stu = (struct Student *)malloc(sizeof(struct Student)); // 动态分配内存
sprintf(stu->id, "S%02d", i + 1); // 学号格式为S01、S02、S03...
sprintf(stu->name, "Student%d", i + 1); // 姓名格式为Student1、Student2、Student3...
for (int j = 0; j < 3; j++) {
stu->score[j] = 0; // 成绩初始值为0
}
stu->next = NULL;
if (head == NULL) {
head = stu;
tail = stu;
} else {
tail->next = stu;
tail = stu;
}
}
return head;
}
// 显示学生链表数据
void show(struct Student *head) {
printf("学号\t姓名\t成绩1\t成绩2\t成绩3\n");
struct Student *p = head;
while (p != NULL) {
printf("%s\t%s\t%.1f\t%.1f\t%.1f\n", p->id, p->name, p->score[0], p->score[1], p->score[2]);
p = p->next;
}
}
// 查找学生数据
void find(struct Student *head) {
char id[10];
printf("请输入要查找的学生学号:");
scanf("%s", id);
struct Student *p = head;
while (p != NULL) {
if (strcmp(p->id, id) == 0) {
printf("学号\t姓名\t成绩1\t成绩2\t成绩3\n");
printf("%s\t%s\t%.1f\t%.1f\t%.1f\n", p->id, p->name, p->score[0], p->score[1], p->score[2]);
return;
}
p = p->next;
}
printf("未找到该学生数据!\n");
}
// 删除符合某个条件的所有学生数据
void del(struct Student **head) {
double minScore;
printf("请输入要删除的学生最低总成绩:");
scanf("%lf", &minScore);
int count = 0;
struct Student *p = *head;
struct Student *pre = NULL;
while (p != NULL) {
double totalScore = p->score[0] + p->score[1] + p->score[2];
if (totalScore < minScore) {
if (pre == NULL) {
*head = p->next; // 删除头结点
} else {
pre->next = p->next; // 删除非头结点
}
free(p); // 释放内存
p = pre == NULL ? *head : pre->next;
count++;
} else {
pre = p;
p = p->next;
}
}
printf("成功删除%d条学生数据!\n", count);
}
// 插入学生数据
void insert(struct Student **head) {
struct Student *newStu = (struct Student *)malloc(sizeof(struct Student)); // 动态分配内存
printf("请输入新学生学号:");
scanf("%s", newStu->id);
printf("请输入新学生姓名:");
scanf("%s", newStu->name);
printf("请输入新学生3门课程成绩:");
scanf("%lf %lf %lf", &newStu->score[0], &newStu->score[1], &newStu->score[2]);
newStu->next = NULL;
if (*head == NULL) {
*head = newStu;
} else {
struct Student *p = *head;
while (p->next != NULL) {
p = p->next;
}
p->next = newStu;
}
printf("成功插入1条学生数据!\n");
}
// 降序排序
void sort(struct Student **head) {
struct Student *p = *head;
struct Student *pre = NULL;
while (p != NULL && p->next != NULL) {
struct Student *q = *head;
struct Student *pre_q = NULL;
while (q != NULL && q->next != NULL) {
double totalScore1 = q->score[0] + q->score[1] + q->score[2];
double totalScore2 = q->next->score[0] + q->next->score[1] + q->next->score[2];
if (totalScore1 < totalScore2 || (totalScore1 == totalScore2 && q->score[0] < q->next->score[0])) {
struct Student *temp = q->next;
q->next = q->next->next;
temp->next = q;
if (pre_q == NULL) {
*head = temp;
} else {
pre_q->next = temp;
}
if (pre != NULL) {
pre->next = temp;
}
pre = temp;
} else {
pre_q = q;
q = q->next;
}
}
}
}
int main() {
struct Student *head = init(); // 初始化学生链表
show(head); // 显示学生链表数据
find(head); // 查找学生数据
del(&head); // 删除符合某个条件的所有学生数据
insert(&head); // 插入学生数据
sort(&head); // 降序排序
show(head); // 显示排序后的学生链表数据
return 0;
}
```
注意:在使用链表进行优化时,需要注意内存的分配和释放,以及对链表头指针的操作。此外,对于删除操作,需要同时记录前一个节点和当前节点,以便删除当前节点时更新前一个节点的指针。
相关推荐
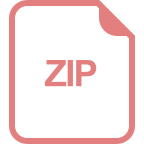
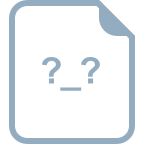
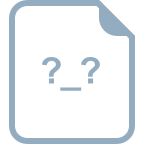














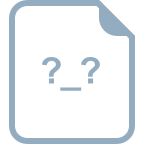