创建一个栈类,要求实现构造函数、判断栈是否为空、出栈、入栈等操作,通过数组来间接实现。使用using namespace std,写一个完整代码
时间: 2024-10-12 11:06:59 浏览: 0
创建一个栈类(Stack),我们可以使用数组作为底层数据结构。这里是一个简单的C++实现:
```cpp
#include <iostream>
using namespace std;
const int DEFAULT_CAPACITY = 50; // 栈的默认容量
class Stack {
private:
int top; // 栈顶指针
int capacity;
int* arr; // 存储栈元素的数组
public:
// 构造函数,初始化栈为空
Stack(int size = DEFAULT_CAPACITY) {
capacity = size;
arr = new int[capacity];
top = -1;
}
// 判断栈是否为空
bool isEmpty() {
return (top == -1);
}
// 入栈操作
void push(int value) {
if (top == capacity - 1) {
cout << "Stack overflow. No space to push." << endl;
return;
}
arr[++top] = value;
cout << "Pushed " << value << " into the stack." << endl;
}
// 出栈操作
int pop() {
if (isEmpty()) {
cout << "Stack is empty. Cannot pop." << endl;
return -1;
}
int poppedValue = arr[top--];
delete[] arr;
arr = new int[capacity]; // 防止内存泄露,每次出栈都重新分配空间
return poppedValue;
}
// 显示栈内容
void display() {
if (isEmpty()) {
cout << "Stack is empty." << endl;
} else {
for (int i = top; i >= 0; i--) {
cout << arr[i] << " ";
}
cout << endl;
}
}
// 销毁栈,释放内存
~Stack() {
delete[] arr;
}
};
int main() {
Stack myStack;
myStack.push(10);
myStack.push(20);
myStack.display(); // 输出: 20 10
cout << "Popped: " << myStack.pop() << endl; // 输出: Popped: 10
myStack.display(); // 输出: 20
return 0;
}
```
相关推荐
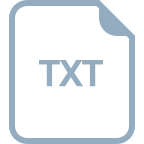
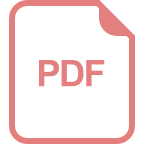
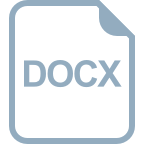














