C++用顺序栈或链栈实现将一个十进制数N转换为r(r=2,8,16)进制数。
时间: 2024-10-14 09:03:21 浏览: 29
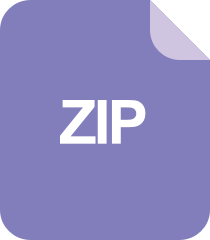
顺序栈、链栈将10进制转为2、8、16进制源码

在C++中,我们可以选择顺序栈(数组实现)或链栈(动态节点实现)来转换十进制数到其他进制。这里我会给出两种方法的简单示例:
**1. 顺序栈实现(数组)**
首先,创建一个栈存储每位数字,并计算每一位直到得到结果。假设我们有一个临时变量用于当前的二进制、八进制或十六进制值。
```cpp
#include <iostream>
#include <stack>
#include <string>
std::string decimalToBase(int N, int base) {
std::stack<char> stack;
while (N > 0) {
char digit = '0' + N % base; // 计算余数对应的字符
stack.push(digit);
N /= base;
}
std::string result = "";
while (!stack.empty()) {
result += stack.top();
stack.pop();
}
return result;
}
int main() {
int N = 12345;
std::cout << "Decimal to Hex: " << decimalToBase(N, 16) << "\n";
return 0;
}
```
**2. 链栈实现(链表)**
如果想要更高效地管理大小未知的数据,可以使用动态结构,比如链表。这里可以创建一个Node结构体,每个节点包含一个字符和指向下一个节点的指针。
```cpp
#include <iostream>
#include <list>
// 定义链表节点
struct Node {
char digit;
Node* next;
};
void decimalToBaseRecursive(Node** head, int N, int base) {
if (N == 0) {
*head = nullptr;
return;
}
Node* newNode = new Node{ '0' + N % base };
newNode->next = *head;
*head = newNode;
decimalToBaseRecursive(&newNode->next, N / base, base);
}
std::string decimalToBaseLinkedList(int N, int base) {
Node* head = nullptr;
decimalToBaseRecursive(&head, N, base);
std::string result;
for (Node* node = head; node != nullptr; node = node->next) {
result += node->digit;
}
delete(head); // 释放内存
return result;
}
int main() {
int N = 12345;
std::cout << "Decimal to Hex (Linked List): " << decimalToBaseLinkedList(N, 16) << "\n";
return 0;
}
```
阅读全文
相关推荐
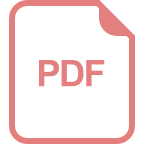
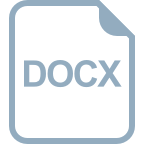
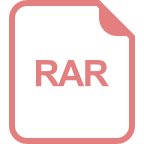
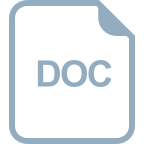
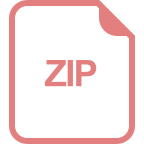
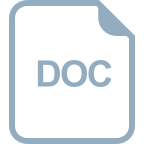
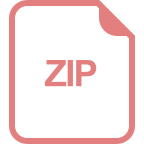
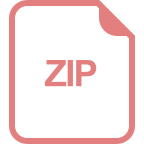
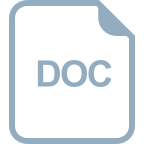
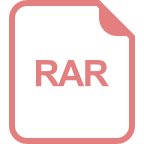
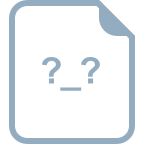




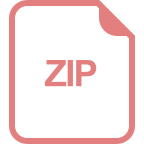