用链栈实现八进制转换十进制
时间: 2023-06-15 21:07:58 浏览: 49
下面是用链栈实现八进制转换十进制的代码实现,具体注释在代码中:
```c++
#include <iostream>
#include <cmath>
using namespace std;
// 定义链栈结构体
struct Node {
int data;
Node* next;
};
// 初始化链栈
void InitStack(Node*& S) {
S = new Node;
S->next = NULL;
}
// 判断链栈是否为空
bool StackEmpty(Node* S) {
return S->next == NULL;
}
// 入栈
void Push(Node*& S, int x) {
Node* p = new Node;
p->data = x;
p->next = S->next;
S->next = p;
}
// 出栈
bool Pop(Node*& S, int& x) {
if (StackEmpty(S)) {
return false;
}
Node* p = S->next;
x = p->data;
S->next = p->next;
delete p;
return true;
}
// 读栈顶元素
bool GetTop(Node* S, int& x) {
if (StackEmpty(S)) {
return false;
}
x = S->next->data;
return true;
}
// 八进制转换十进制
int OctToDec(char s[]) {
Node* S = NULL;
InitStack(S);
int i = 0;
while (s[i] != '\0') {
Push(S, s[i] - '0'); // 将字符转换为数字并入栈
i++;
}
int dec = 0;
int n = 0;
int x;
while (!StackEmpty(S)) {
Pop(S, x);
dec += x * pow(8, n); // 按位计算
n++;
}
return dec;
}
int main() {
char s[100];
cout << "请输入一个八进制数:";
cin >> s;
int dec = OctToDec(s);
cout << "转换为十进制为:" << dec << endl;
return 0;
}
```
这里使用了链栈来实现,具体的实现过程如下:
1. 从字符串的最后一位开始遍历,将每一位的数字转换为整数,并入栈。
2. 然后按照栈的后进先出的顺序,将每一位数字取出,并按照位数计算十进制值。
3. 最后得到的十进制值即为所求。
这里使用了 pow 函数来计算 $8^n$,需要在头文件中添加 math.h。
相关推荐
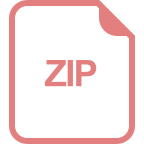














