用c语言的链栈实现十进制转换成十六进制
时间: 2023-09-11 16:04:18 浏览: 63
好的,我可以为您解答这个问题。
首先,我们需要定义一个链栈的结构体,其中包括一个指向栈顶节点的指针,以及链栈的长度:
```c
typedef struct node {
int data;
struct node *next;
} Node;
typedef struct {
Node *top;
int len;
} Stack;
```
接下来,我们可以编写一个函数,将十进制数转换成十六进制数并压入链栈中:
```c
void dec2hex(Stack *s, int dec) {
int rem;
while (dec > 0) {
rem = dec % 16;
push(s, rem);
dec /= 16;
}
}
```
其中,`push()` 函数用于将元素压入链栈中:
```c
void push(Stack *s, int data) {
Node *new_node = (Node*)malloc(sizeof(Node));
new_node->data = data;
new_node->next = s->top;
s->top = new_node;
s->len++;
}
```
最后,我们可以编写一个函数,将链栈中的元素依次弹出并输出,即可得到最终的十六进制数:
```c
void print_hex(Stack *s) {
Node *p = s->top;
while (p != NULL) {
if (p->data < 10) {
printf("%d", p->data);
} else {
printf("%c", 'A' + p->data - 10);
}
p = p->next;
}
}
```
完整的代码如下所示:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct node {
int data;
struct node *next;
} Node;
typedef struct {
Node *top;
int len;
} Stack;
void push(Stack *s, int data) {
Node *new_node = (Node*)malloc(sizeof(Node));
new_node->data = data;
new_node->next = s->top;
s->top = new_node;
s->len++;
}
void dec2hex(Stack *s, int dec) {
int rem;
while (dec > 0) {
rem = dec % 16;
push(s, rem);
dec /= 16;
}
}
void print_hex(Stack *s) {
Node *p = s->top;
while (p != NULL) {
if (p->data < 10) {
printf("%d", p->data);
} else {
printf("%c", 'A' + p->data - 10);
}
p = p->next;
}
}
int main() {
int dec;
Stack s = {NULL, 0};
printf("请输入一个十进制数:");
scanf("%d", &dec);
dec2hex(&s, dec);
printf("该数的十六进制表示为:");
print_hex(&s);
printf("\n");
return 0;
}
```
当然,这只是其中的一种实现方式,您也可以根据自己的需求进行调整。
相关推荐
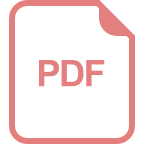














