利用链栈的基本操作,设计一个算法将一个非负十进制数转换成一个十六进制数数字串并输出。
时间: 2023-06-13 09:08:49 浏览: 107
算法步骤如下:
1. 初始化一个空的链栈;
2. 对于给定的非负十进制数,不断将其除以16,将每次的余数压入链栈中,直至商为0;
3. 从链栈底部开始依次弹出每个余数,根据十六进制规则转换成相应的数字或字母,并输出,直至链栈为空。
以下是算法的具体实现(使用C语言):
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct Node {
int data; // 存储余数
struct Node *next; // 指向下一个节点的指针
} Node;
typedef struct Stack {
Node *bottom; // 链栈底部指针
Node *top; // 链栈顶部指针
} Stack;
// 初始化链栈
void initStack(Stack *s) {
s->bottom = (Node *)malloc(sizeof(Node));
s->top = s->bottom;
s->top->next = NULL;
}
// 判断链栈是否为空
int isEmpty(Stack *s) {
return s->bottom == s->top;
}
// 入栈
void push(Stack *s, int x) {
Node *new_node = (Node *)malloc(sizeof(Node));
new_node->data = x;
new_node->next = s->top;
s->top = new_node;
}
// 出栈
int pop(Stack *s) {
if(isEmpty(s)) {
printf("Stack is empty.\n");
return -1;
}
int x = s->top->data;
Node *to_delete = s->top;
s->top = s->top->next;
free(to_delete);
return x;
}
// 十进制转十六进制
void decimalToHex(Stack *s, int decimal) {
while(decimal > 0) {
int remainder = decimal % 16;
push(s, remainder);
decimal /= 16;
}
while(!isEmpty(s)) {
int remainder = pop(s);
if(remainder >= 0 && remainder <= 9) {
printf("%d", remainder);
} else {
printf("%c", remainder - 10 + 'A');
}
}
}
int main() {
int decimal;
printf("Please input a non-negative decimal integer: ");
scanf("%d", &decimal);
Stack s;
initStack(&s);
printf("The corresponding hexadecimal number is: ");
decimalToHex(&s, decimal);
printf("\n");
return 0;
}
```
阅读全文
相关推荐
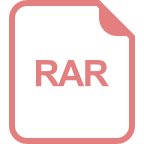
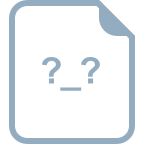
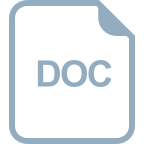
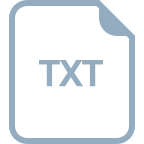
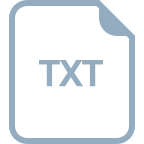
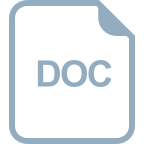
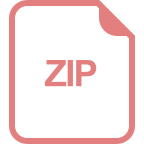
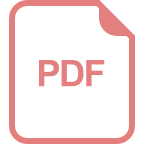
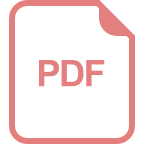
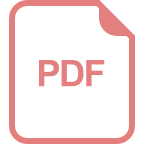
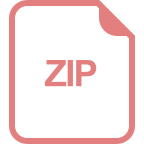
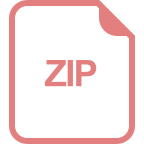
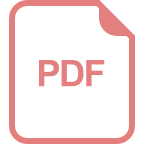
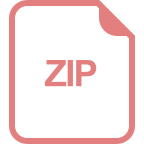
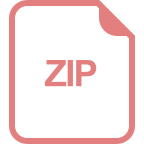
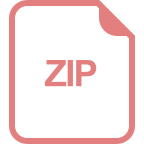
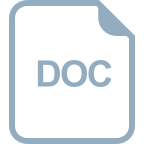