定义一个复数类complex,建立一个Complex对象并调用
时间: 2024-09-13 20:14:48 浏览: 42
在面向对象编程中,定义一个复数类(Complex)通常需要包含复数的实部(real part)和虚部(imaginary part),以及对复数进行操作的方法,例如加法、减法、乘法、除法等。以下是一个简单的复数类的定义以及如何创建一个Complex对象并进行基本操作的示例:
```java
public class Complex {
private double real; // 实部
private double imaginary; // 虚部
// 构造函数
public Complex(double real, double imaginary) {
this.real = real;
this.imaginary = imaginary;
}
// 获取实部
public double getReal() {
return real;
}
// 设置实部
public void setReal(double real) {
this.real = real;
}
// 获取虚部
public double getImaginary() {
return imaginary;
}
// 设置虚部
public void setImaginary(double imaginary) {
this.imaginary = imaginary;
}
// 加法操作
public Complex add(Complex other) {
return new Complex(this.real + other.real, this.imaginary + other.imaginary);
}
// 减法操作
public Complex subtract(Complex other) {
return new Complex(this.real - other.real, this.imaginary - other.imaginary);
}
// 乘法操作
public Complex multiply(Complex other) {
double newReal = this.real * other.real - this.imaginary * other.imaginary;
double newImaginary = this.real * other.imaginary + this.imaginary * other.real;
return new Complex(newReal, newImaginary);
}
// 除法操作
public Complex divide(Complex other) {
double denominator = other.real * other.real + other.imaginary * other.imaginary;
double newReal = (this.real * other.real + this.imaginary * other.imaginary) / denominator;
double newImaginary = (this.imaginary * other.real - this.real * other.imaginary) / denominator;
return new Complex(newReal, newImaginary);
}
// 字符串表示
@Override
public String toString() {
return "(" + real + " + " + imaginary + "i)";
}
}
// 使用Complex类
public class ComplexTest {
public static void main(String[] args) {
Complex c1 = new Complex(3.0, 4.0); // 创建Complex对象c1
Complex c2 = new Complex(1.0, -2.0); // 创建Complex对象c2
// 调用复数对象的方法
Complex sum = c1.add(c2); // c1加c2
Complex difference = c1.subtract(c2); // c1减c2
Complex product = c1.multiply(c2); // c1乘c2
Complex quotient = c1.divide(c2); // c1除c2
// 打印结果
System.out.println("Sum: " + sum);
System.out.println("Difference: " + difference);
System.out.println("Product: " + product);
System.out.println("Quotient: " + quotient);
}
}
```
在这个例子中,我们定义了一个复数类`Complex`,它有两个属性`real`和`imaginary`,以及几个基本操作的方法。然后在`ComplexTest`类的`main`方法中,我们创建了两个`Complex`对象,并对它们执行了加、减、乘、除运算,最后将结果打印到控制台。
阅读全文
相关推荐
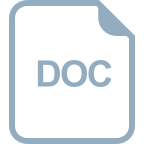
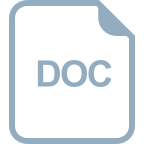
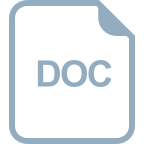















