用c语言解决炼金配方问题
时间: 2024-10-28 20:12:59 浏览: 4
在C语言中解决炼金配方问题,通常可以采用数组、结构体或者关联数据结构如哈希表来存储配方信息。假设我们有多个原料对应一种产品,可以设计如下的基本框架:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义一个配方结构体
typedef struct {
char product[50]; // 产品名称
int ingredients[50][2]; // 存放原料及用量,如 {原料名, 数量}
int num_ingredients; // 原料数量
} Recipe;
// 添加新配方到列表
void add_recipe(Recipe *recipes, char *product, int ingredient_data[], int num) {
recipes->num_ingredients = num;
for (int i = 0; i < num; i++) {
strcpy(recipes->ingredients[i], ingredient_data[i*2]);
recipes->ingredients[i][strlen(recipes->ingredients[i])] = '\0';
recipes->ingredients[i+num] = ingredient_data[i*2+1];
}
}
// 检查是否能通过已有的配方合成目标产品
int can_produce(char target[], Recipe *recipes) {
for (int i = 0; i < recipes->num_ingredients; i += 2) {
if (!strcmp(target, recipes->ingredients[i])) {
return recipes->ingredients[i+1]; // 返回所需原料数量
}
}
return -1; // 如果找不到匹配的原料,则返回-1表示无法生产
}
int main() {
Recipe recipes[] = {{"魔法药水", {"魔草", 2}, {"水", 3}}, ...}; // 初始化更多配方
// 使用示例
char target_product[] = "魔法药水";
int needed_material = can_produce(target_product, recipes);
if (needed_material != -1)
printf("需要 %d 份%s才能制作魔法药水.\n", needed_material, target_product);
return 0;
}
阅读全文
相关推荐
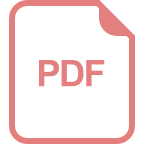
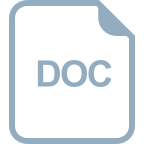
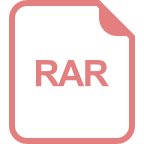
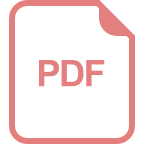