第一题:上传多个文件后显示文件列表,点击后能下载,并且有如下限制:每个文件不能超过3M字节,每次上传总的上传文件不能超过10M字节,并且只能上传pdf类型的文件。用springboot的全部代码
时间: 2024-11-12 12:40:54 浏览: 5
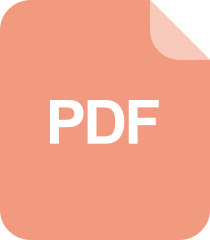
PHP文件操作实例总结【文件上传、下载、分页】
在Spring Boot项目中实现这样的功能,我们需要结合Web MVC、文件上传处理以及一些验证策略。以下是一个简化版的示例代码,展示了关键部分:
```java
import org.springframework.beans.factory.annotation.Value;
import org.springframework.core.io.FileSystemResource;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.multipart.MultipartFile;
import java.io.IOException;
import java.util.List;
@Controller
public class FileUploadController {
@Value("${file.upload.path}")
private String uploadPath;
@PostMapping("/upload")
public ResponseEntity<List<FileDetails>> handleFileUpload(@RequestParam("files") List<MultipartFile> files) {
if (files == null || files.isEmpty()) {
return new ResponseEntity<>(HttpStatus.BAD_REQUEST);
}
// 检查总大小是否超过10MB
long totalSize = files.stream().mapToLong(MultipartFile::getSize).sum();
if (totalSize > 10 * 1024 * 1024) {
return new ResponseEntity<>("Total file size exceeds limit", HttpStatus.BAD_REQUEST);
}
// 检查单个文件大小和类型
List<FileDetails> details = files.stream()
.filter(file -> file.getSize() <= 3 * 1024 * 1024 && isPdfType(file))
.map(this::processFile)
.collect(Collectors.toList());
return ResponseEntity.ok(details);
}
private boolean isPdfType(MultipartFile file) {
String filename = file.getOriginalFilename();
return filename.endsWith(".pdf");
}
private FileDetails processFile(MultipartFile file) throws IOException {
FileSystemResource resource = new FileSystemResource(new File(uploadPath + "/" + file.getOriginalFilename()));
return new FileDetails(file.getOriginalFilename(), resource.getFile().length());
}
// 文件详情实体类
private static class FileDetails {
private String fileName;
private long fileSize;
// 构造函数、getter/setter...
}
}
```
注意,这只是一个基础的实现,实际应用中还需要考虑异常处理、日志记录等其他细节。同时,`@Value("${file.upload.path}")`需要在配置文件application.properties中设置具体的上传路径。
阅读全文
相关推荐
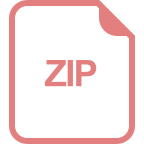
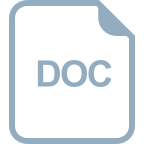


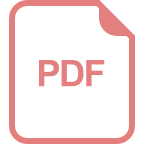
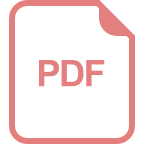
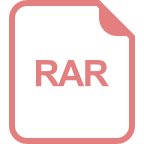
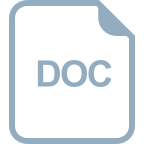
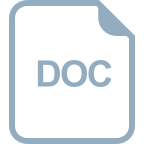
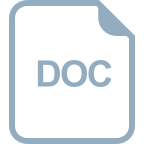
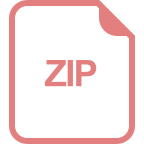
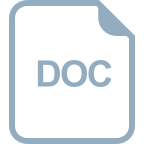
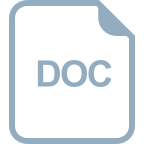
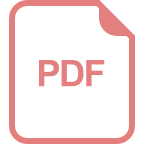



