b. Write a linked list implementation of self-adjusting lists. by c language
时间: 2024-10-03 17:03:11 浏览: 40
自调整列表(Adaptive Array List),也称为动态数组,是一种数据结构,它结合了链表和数组的优点,能自动调整存储容量以适应元素的增删。在C语言中,我们可以创建一个简单的双向链表实现自调整列表:
```c
#include <stdlib.h>
typedef struct Node {
int data;
struct Node* prev;
struct Node* next;
} Node;
typedef struct AdaptiveList {
Node* head;
Node* tail;
int size;
int capacity;
} AdaptiveList;
// 初始化列表
AdaptiveList* init_list() {
AdaptiveList* list = (AdaptiveList*)malloc(sizeof(AdaptiveList));
list->head = NULL;
list->tail = NULL;
list->size = 0;
list->capacity = 4; // 初始容量
return list;
}
// 插入元素到列表尾部
void insert(AdaptiveList* list, int value) {
if ((list->size + 1) >= list->capacity) {
resize_list(list); // 自动扩容
}
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = value;
newNode->prev = list->tail;
newNode->next = NULL;
if (list->tail != NULL) {
list->tail->next = newNode;
} else {
list->head = newNode;
}
list->tail = newNode;
list->size++;
}
// 扩容函数
void resize_list(AdaptiveList* list) {
list->capacity *= 2;
Node* newTail = (Node*)malloc(sizeof(Node) * list->capacity);
newTail->prev = list->tail;
newTail->next = NULL;
if (list->tail != NULL) {
list->tail->next = newTail;
} else {
list->head = newTail;
}
list->tail = newTail;
}
// 删除指定位置的元素
void delete_at(AdaptiveList* list, int index) {
if (index >= 0 && index < list->size) {
if (index == 0) {
if (list->head == list->tail) {
free(list->head);
list->head = NULL;
list->tail = NULL;
} else {
list->head = list->head->next;
list->head->prev = NULL;
}
} else {
Node* current = list->head;
for (int i = 0; i < index - 1; i++) {
current = current->next;
}
current->next = current->next->next;
if (current->next != NULL) {
current->next->prev = current;
}
}
list->size--;
}
}
// 示例操作
void main_example(AdaptiveList* list) {
init_list();
insert(list, 1);
insert(list, 2);
delete_at(list, 0);
printf("Size: %d, Capacity: %d\n", list->size, list->capacity);
}
阅读全文
相关推荐










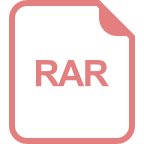







