1. The following code implements a linked-list Node: struct Node { int data; Node *next; }; Node *A; a) Write a new function to delete the Nth element in the linked-list. The new function's prototype is: bool DeleteNthNode(Node * & listpointer, int nth_element); If the deletion is completed, the function should return true. If the linked-list has less than N elements, then the function should return false. Make sure that your code covers all cases: when the list is empty, when the list only has one element, or two elements etc.
时间: 2024-03-06 12:52:20 浏览: 53
Here's an implementation of the DeleteNthNode function:
```
bool DeleteNthNode(Node *&listpointer, int nth_element) {
// Check if the list is empty
if (listpointer == NULL) {
return false;
}
// Check if the first node needs to be deleted
if (nth_element == 1) {
Node *temp = listpointer;
listpointer = listpointer->next;
delete temp;
return true;
}
// Traverse the list to find the node before the nth node
Node *prev = NULL;
Node *curr = listpointer;
int count = 1;
while (count < nth_element && curr != NULL) {
prev = curr;
curr = curr->next;
count++;
}
// Check if the nth node was found
if (curr == NULL) {
return false;
}
// Delete the nth node
prev->next = curr->next;
delete curr;
return true;
}
```
This implementation checks for all the cases mentioned in the prompt - empty list, deleting the first node, and deleting a node in the middle of the list. The function takes a reference to the listpointer so that it can modify the list if necessary.
阅读全文
相关推荐
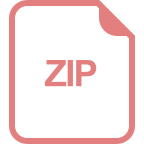
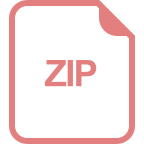
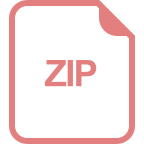
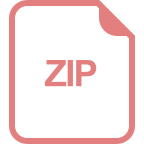
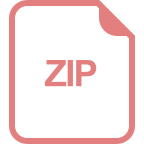
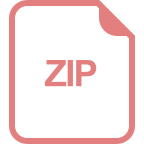
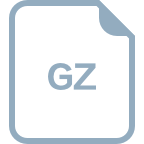
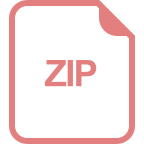
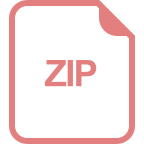
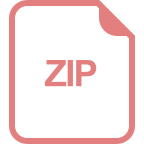
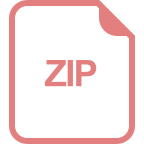
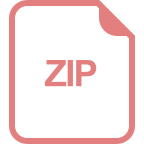
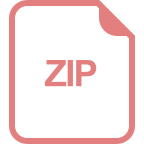
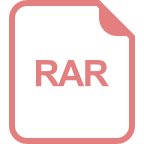
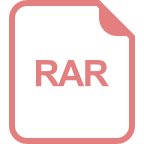
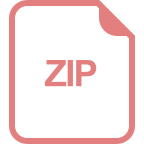
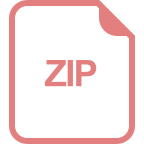
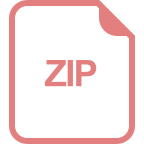
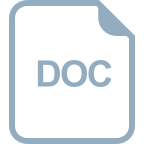