java用循环单链表结构设计队列
时间: 2023-12-07 08:37:13 浏览: 112
使用循环单链表结构设计队列,需要定义一个Node类来表示链表中的节点,以及一个Queue类来表示队列。具体实现步骤如下:
1.定义Node类,包含一个数据域和一个指向下一个节点的指针域。
2.定义Queue类,包含一个头指针和一个尾指针,以及队列的大小。
3.实现队列的入队操作,即在队尾插入一个元素。如果队列为空,则将头指针和尾指针都指向新节点;否则,将尾指针指向新节点,并将新节点的next指针指向头节点。
4.实现队列的出队操作,即删除队头元素。如果队列为空,则返回null;否则,将头指针指向下一个节点,并返回删除的节点的数据域。
5.实现队列的判空操作,即判断头指针是否为空。
6.实现队列的获取队头元素操作,即返回头指针指向的节点的数据域。
以下是Java代码实现:
```java
class Node {
int data;
Node next;
public Node(int data) {
this.data = data;
this.next = null;
}
}
class Queue {
Node head;
Node tail;
int size;
public Queue() {
head = null;
tail = null;
size = 0;
}
public void enqueue(int data) {
Node newNode = new Node(data);
if (head == null) {
head = newNode;
tail = newNode;
tail.next = head;
} else {
tail.next = newNode;
tail = newNode;
tail.next = head;
}
size++;
}
public int dequeue() {
if (head == null) {
return -1;
} else {
int data = head.data;
head = head.next;
tail.next = head;
size--;
return data;
}
}
public boolean isEmpty() {
return head == null;
}
public int getFront() {
if (head == null) {
return -1;
} else {
return head.data;
}
}
}
```
阅读全文
相关推荐
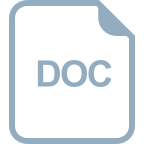
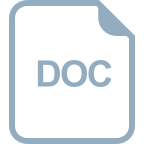
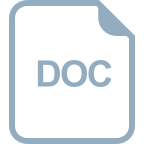

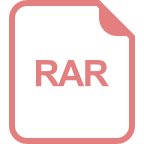
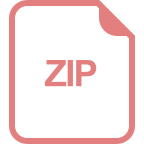
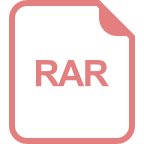
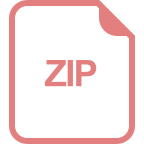
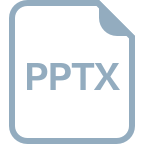
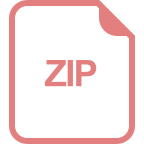
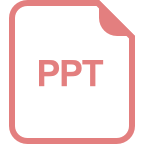
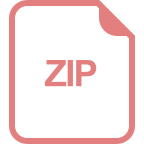
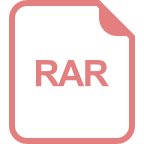
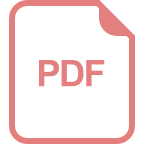
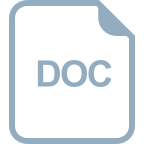
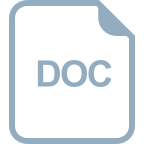
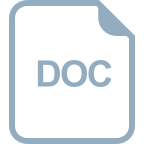
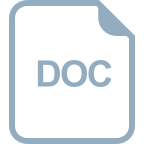
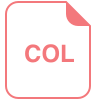