python tuple的遍历
时间: 2024-11-29 20:09:30 浏览: 6
在Python中,遍历tuple(元组)与遍历列表类似,但是由于元组是不可变的,你不能像修改列表那样修改元组中的元素。以下是遍历tuple的基本方法:
1. 使用for循环遍历:
```python
my_tuple = (1, 'two', 3.0, True)
for item in my_tuple:
print(item)
```
这会依次打印出元组中的每个元素。
2. 遍历键值对(如果元组包含二元组或字典推导式形成的元组):
```python
my_tuple = ((1, 'one'), ('two', 2), ('three', 3))
for pair in my_tuple:
key, value = pair # 分解元组
print(f"Key: {key}, Value: {value}")
```
这里假设元组内的元素是有序的键值对。
3. 使用enumerate()函数同时获取索引和元素:
```python
for index, value in enumerate(my_tuple):
print(f"Element at index {index}: {value}")
```
这将显示元组中每个元素的索引及其对应的值。
相关问题
python 遍历tuple
遍历Python中的tuple有多种方法。你可以使用for循环来遍历tuple中的每个元素,也可以使用enumerate()函数获得索引和值,或者使用range()函数和len()函数结合来遍历tuple。另外,你还可以使用迭代器iter()来遍历tuple的元素。
以下是几种遍历tuple的方法:
1. 使用for循环遍历tuple的每个元素:
```python
for value in tuple:
print(value)
```
结果:
```
111
222
333
```
2. 使用enumerate()函数获得索引和值:
```python
for index, value in enumerate(tuple):
print('index:', index, 'value:', value)
```
结果:
```
index: 0 value: 111
index: 1 value: 222
index: 2 value: 333
```
3. 使用range()函数和len()函数结合遍历tuple:
```python
for index in range(len(tuple)):
print('index:', index, 'value:', tuple[index])
```
结果:
```
index: 0 value: 111
index: 1 value: 222
index: 2 value: 333
```
4. 使用迭代器iter()遍历tuple的元素:
```python
for value in iter(tuple):
print(value)
```
结果:
```
111
222
333
```
python怎样遍历数值
在Python中,遍历数值可以有多种方式,取决于你要操作的是哪种类型的数值数据结构。以下是几种常见的遍历:
1. **列表(List)**:使用`for`循环简单遍历每个元素。
```python
numbers = [1, 2, 3, 4, 5]
for num in numbers:
print(num)
```
2. **元组(Tuple)**:同理,也可以遍历元组中的每个元素。
```python
tup = (6, 7, 8, 9, 10)
for item in tup:
print(item)
```
3. **字符串(String)**:字符串被视为字符序列,同样可以用`for`循环遍历。
```python
s = 'abcdefg'
for char in s:
print(char)
```
4. **集合(Set)**:虽然集合不保证顺序,但可以通过迭代器遍历元素。
```python
my_set = {11, 12, 13}
for value in my_set:
print(value)
```
5. **字典(Dictionary)**:需要通过键(key)来访问值,可以使用`for`循环配合`items()`方法。
```python
my_dict = {'a': 1, 'b': 2, 'c': 3}
for key, value in my_dict.items():
print(f'{key}: {value}')
```
6. **range() 函数**:用于生成数字序列,常用于控制循环次数。
```python
for i in range(1, 6):
print(i)
```
阅读全文
相关推荐
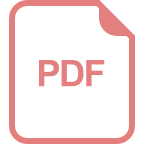
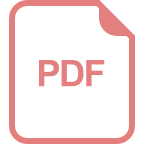
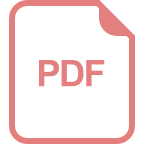













