unordered_map+map
时间: 2023-11-11 11:05:33 浏览: 47
unordered_map和map都是C++ STL中的关联容器,它们都可以用于存储键值对。但是它们的底层实现不同,map底层是红黑树,而unordered_map底层是哈希表。因此,它们在插入、查找、删除等操作上的性能表现也不同。如果需要快速的查找和插入操作,可以选择unordered_map,如果需要有序的键值对,可以选择map。
关于unordered_map的使用,可以使用insert()函数插入键值对,使用find()函数查找键对应的值,使用erase()函数删除键值对。同时,unordered_map也支持迭代器,可以使用begin()和end()函数获取迭代器,使用*运算符获取键值对。
<<相关问题>>
1. map和unordered_map的底层实现分别是什么?
2. unordered_set和set的区别是什么?
3. 如何遍历unordered_map中的所有键值对?
相关问题
unordered_map+自定义结构体
unordered_map是C++标准库中的容器,用于存储键-值对并提供快速的查找功能。对于自定义结构体,可以使用unordered_map来存储该结构体作为键,并指定自定义的哈希函数和键的比较规则。
有三种常见的方式可以实现这个目标:
方法一:将自定义结构体中重载operator == 和自定义hash函数,并在unordered_map中传入自定义结构体类型和自定义hash函数类型,不传入第四个参数。
```cpp
#include <iostream>
#include <unordered_map>
#include <functional>
class Person {
public:
int _age;
Person(int age = -1) :_age(age) {}
bool operator == (const Person& p) const {
return _age == p._age;
}
};
struct PersonHash {
public:
size_t operator()(const Person& p) const {
return std::hash<int>()(p._age);
}
};
int main() {
std::unordered_map<Person, int, PersonHash> um;
um.insert(std::make_pair<Person, int>(Person(1), 1));
um.insert(std::make_pair<Person, int>(Person(2), 1));
for (auto& e : um) {
std::cout << e.first._age << " " << e.second << std::endl;
}
return 0;
}
```
方法二:自定义hash函数和自定义键的比较规则,并在unordered_map中传入自定义结构体类型、自定义hash函数类型和自定义键的比较规则类型。
```cpp
#include <iostream>
#include <unordered_map>
#include <functional>
class Person {
public:
int _age;
Person(int age = -1) :_age(age) {}
};
struct PersonHash {
public:
size_t operator()(const Person& p) const {
return std::hash<int>()(p._age);
}
};
struct PersonEqual {
public:
bool operator()(const Person& p1, const Person& p2) const {
return p1._age == p2._age;
}
};
int main() {
std::unordered_map<Person, int, PersonHash, PersonEqual> um;
um.insert(std::make_pair<Person, int>(Person(1), 1));
um.insert(std::make_pair<Person, int>(Person(2), 1));
for (auto& e : um) {
std::cout << e.first._age << " " << e.second << std::endl;
}
return 0;
}
```
方法三:传入自定义结构体类型,不重载operator == 和自定义hash函数。
```cpp
#include <iostream>
#include <unordered_map>
class Person {
public:
int _age;
Person(int age = -1) :_age(age) {}
};
int main() {
std::unordered_map<Person, int> um;
um.insert(std::make_pair<Person, int>(Person(1), 1));
um.insert(std::make_pair<Person, int>(Person(2), 1));
for (auto& e : um) {
std::cout << e.first._age << " " << e.second << std::endl;
}
return 0;
}
```
以上是三种使用unordered_map存储自定义结构体的方法,具体使用哪种方式取决于你的需求和喜好。
c+++unordered_map
C++中的unordered_map是一种关联容器,它使用哈希表来存储键值对。与map相比,unordered_map的查找速度更快,因为它的查找时间是常量级别的。但是,unordered_map的建立比较耗费时间。此外,与map不同,unordered_map的遍历顺序与创建该容器时输入元素的顺序是不一定一致的,遍历是按照哈希表从前往后依次遍历的。
下面是一个简单的unordered_map的例子:
```c++
#include <iostream>
#include <unordered_map>
#include <string>
int main() {
std::unordered_map<std::string, int> myMap = {
{"apple", 1},
{"banana", 2},
{"orange", 3}
};
std::cout << "The value of apple is: " << myMap["apple"] << std::endl;
std::cout << "The value of banana is: " << myMap["banana"] << std::endl;
std::cout << "The value of orange is: " << myMap["orange"] << std::endl;
return 0;}
```
输出结果为:
```
The value of apple is: 1
The value of banana is: 2
The value of orange is: 3
```
相关推荐
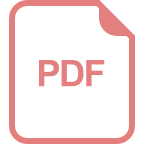
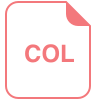
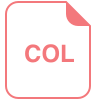
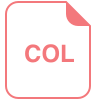
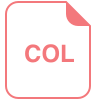
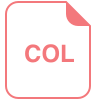







