linux 无名管道代码分析
时间: 2024-09-19 20:17:12 浏览: 40
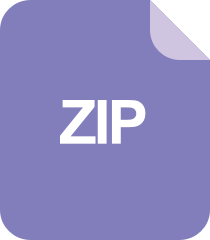
进程通信之无名管道.zip
Linux 无名管道(也称为FIFO,即File In/Out Channel)是一种半双工通信机制,它允许两个进程通过创建一个特殊类型的文件来进行通信,而无需预设的命名路径。这种通信是在内核级进行的,因此效率较高。以下是基本的无名管道操作的代码分析:
```c
#include <stdio.h>
#include <unistd.h>
#include <fcntl.h>
#define READ_END 0
#define WRITE_END 1
int main(int argc, char *argv[]) {
int pipe_fd[2]; // 创建一个管道句柄数组
char message[] = "Hello, Pipe!";
if (pipe(pipe_fd) == -1) { // 如果创建失败,返回错误
perror("Pipe creation failed");
return 1;
}
pid_t child_pid = fork(); // 创建子进程
if (child_pid < 0) {
perror("Fork failed");
return 1;
} else if (child_pid == 0) { // 子进程
close(pipe_fd[WRITE_END]); // 子进程关闭写端
write(pipe_fd[READ_END], message, strlen(message)); // 子进程向管道写入数据
printf("Child process wrote to pipe and exited.\n");
exit(0);
} else { // 父进程
close(pipe_fd[READ_END]); // 父进程关闭读端
char buffer[1024];
read(pipe_fd[WRITE_END], buffer, sizeof(buffer)); // 父进程从管道读取数据
printf("Parent process read from pipe: %s\n", buffer);
wait(NULL); // 等待子进程结束
}
return 0;
}
```
在这个例子中,
- 父进程首先创建了一个管道,并将其两端分别保存在`pipe_fd`数组的`READ_END`和`WRITE_END`位置。
- `fork()`函数用于创建子进程,新生成的子进程会拥有相同的`pipe_fd`副本。
- 子进程负责将消息写入管道(`write`),然后结束。
- 父进程则关闭读端,从管道读取数据(`read`),并打印出来。
阅读全文
相关推荐
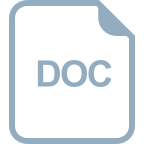
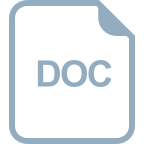
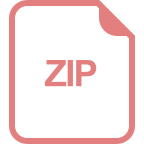

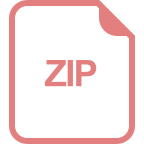
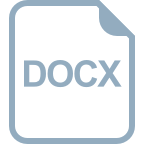
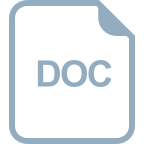
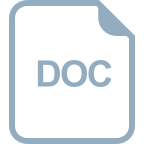
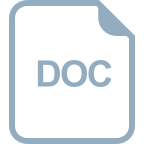



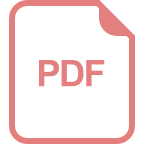
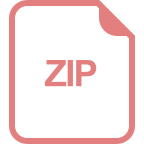
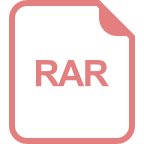
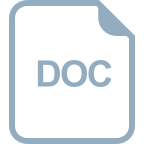

