利用循环队列编程实现一个缓存,具备以下功能:具备缓存的读写功能,并且每缓存五组数据,从缓存一次性读出处理
时间: 2024-10-21 11:07:56 浏览: 31
在编程中,我们可以利用循环队列(Circular Queue)作为缓存结构,它是一个先进先出(FIFO,First In First Out)的数据结构,非常适合用于存储最近使用的数据。以下是利用循环队列实现一个读写缓存并每5组数据批量处理的一个简要步骤:
```python
class Cache:
def __init__(self, capacity):
self.capacity = capacity
# 使用列表模拟循环队列
self.queue = [None] * capacity
self.head = -1 # 队头位置
self.tail = -1 # 队尾位置
def is_empty(self):
return self.head == -1 and self.tail == -1
def enqueue(self, data):
if self.is_full():
self.shift() # 如果满,先删除最旧的数据
self.tail = (self.tail + 1) % self.capacity
self.queue[self.tail] = data
def dequeue(self):
if self.is_empty():
raise IndexError("Cache is empty")
data = self.queue[self.head]
self.head = (self.head + 1) % self.capacity
return data
def shift(self):
while not self.is_empty():
self.dequeue()
def process_batch(self):
batch_size = 5
start = max(0, self.head - batch_size)
end = self.tail if start < batch_size else start + batch_size
processed_data = [self.dequeue() for _ in range(start, end)]
# 这里可以对processed_data进行批量处理...
print(f"Processed {batch_size} items: {processed_data}")
# 使用示例
cache = Cache(capacity=10)
cache.enqueue('A')
cache.enqueue('B')
cache.enqueue('C')
cache.enqueue('D')
cache.enqueue('E') # 此时队列长度为1,因为尾部索引会绕回
cache.enqueue('F') # 队列为 A->B->C->D->E
cache.process_batch() # 输出:Processed 5 items: ['A', 'B', 'C', 'D', 'E']
cache.enqueue('G') # 队列为 G->D->E->F->B
cache.process_batch() # 输出:Processed 5 items: ['D', 'E', 'F', 'B', None] (由于G已满出队)
```
阅读全文
相关推荐
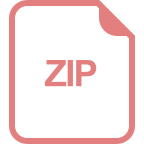
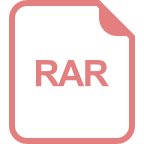
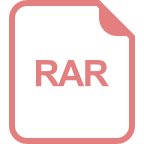







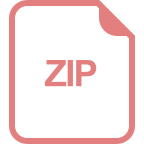
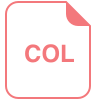
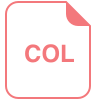
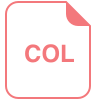
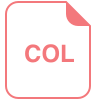
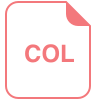
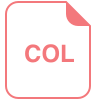
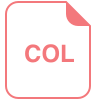
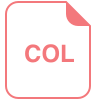