用c++实现RSA算法,其中已知n、e、c,求出m
时间: 2024-09-07 09:02:57 浏览: 66
在C++中实现RSA算法通常涉及密钥生成、加密和解密过程。如果你已知公钥(n, e)和密文(c),想要解密得到原始消息(m),可以按照以下步骤进行:
1. **大数运算库导入**:
首先,你需要一个支持大整数计算的库,如`<openssl>`中的`BN`(Big Number)类。
```cpp
#include <openssl/bio.h>
#include <openssl/buffer.h>
#include <openssl/pem.h>
#include <openssl/rsa.h>
```
2. **加载密钥**:
公钥(n, e)需要从PEM格式读取,并转换成RSA结构。
```cpp
RSA* rsa = RSA_new();
BIO* bioIn = BIO_new_mem_buf((char*)n, -1);
if (!RSA_load_key_bio(rsa, bioIn)) {
// 错误处理
}
```
3. **密文解密**:
使用`RSA_private_decrypt()`函数解密密文。这个函数接受密文、解密模式、padding类型以及用于临时存储结果的buffer。
```cpp
int plaintext_len = RSA_size(rsa); // 获取明文长度
unsigned char* decrypted_msg = new unsigned char[plaintext_len];
int ret = RSA_private_decrypt(c, plaintext_len, decrypted_msg, rsa, RSA_PKCS1_PADDING);
if (ret == -1) {
// 解密失败,错误处理
}
// 把解密后的字符串转换回字符数组或其他形式
std::string m(reinterpret_cast<char*>(decrypted_msg), ret);
delete[] decrypted_msg;
```
4. **清理内存和关闭资源**:
最后别忘了释放RSA和BIO对象,以及处理其他可能的内存分配。
```cpp
RSA_free(rsa);
BIO_free_all(bioIn);
```
阅读全文
相关推荐
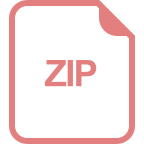
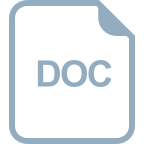
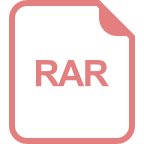

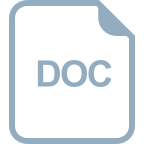
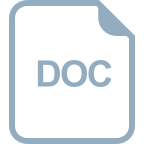
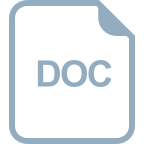
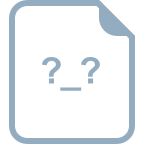
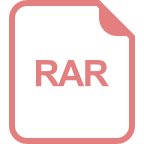
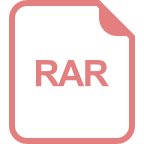
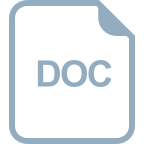
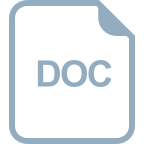
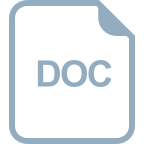
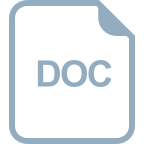
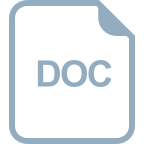



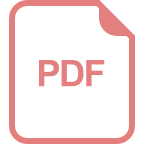