给我一个封装好的把lidar数据投影到图像上的python代码
时间: 2024-04-15 07:30:37 浏览: 80
当然可以!以下是一个简单的示例代码,可以将LiDAR数据投影到图像上:
```python
import numpy as np
import cv2
def project_lidar_to_image(lidar_points, image_width, image_height, fov_horizontal, fov_vertical):
# LiDAR parameters
lidar_width = 100 # Assuming LiDAR covers 100 meters width
lidar_height = 30 # Assuming LiDAR covers 30 meters height
# Calculate scaling factors
scale_x = image_width / lidar_width
scale_y = image_height / lidar_height
# Create blank image
image = np.zeros((image_height, image_width), dtype=np.uint8)
# Project LiDAR points to image
for point in lidar_points:
x, y, z = point
if abs(x) < lidar_width / 2 and abs(y) < lidar_height / 2 and z > 0:
# Convert LiDAR coordinates to image coordinates
img_x = int((x + lidar_width / 2) * scale_x)
img_y = int(image_height - (y + lidar_height / 2) * scale_y)
# Draw projected point on image
cv2.circle(image, (img_x, img_y), 2, (255, 255, 255), -1)
return image
# Example usage
lidar_points = [(10, 5, 2), (20, -10, 5), (-5, 15, 3)] # Sample LiDAR points
image_width = 640
image_height = 480
fov_horizontal = 90
fov_vertical = 60
projected_image = project_lidar_to_image(lidar_points, image_width, image_height, fov_horizontal, fov_vertical)
# Display the projected image
cv2.imshow("Projected Image", projected_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
请注意,这只是一个基本的示例代码,假设LiDAR的覆盖范围为100x30米,并且LiDAR点的坐标为(x, y, z)。您可以根据实际情况进行调整和修改。此代码使用OpenCV库来绘制图像。
希望能对您有所帮助!如果您有任何其他问题,请随时问我。
阅读全文
相关推荐
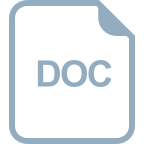
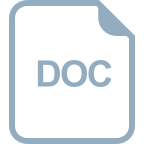
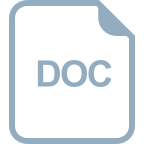
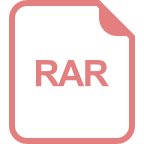
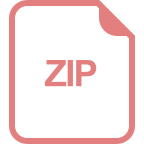

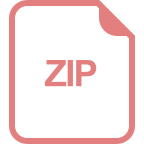
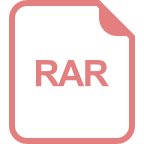
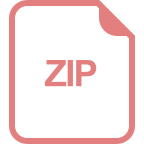
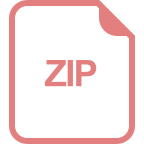
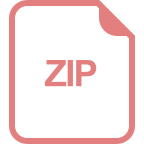
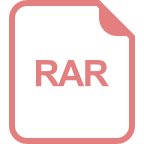
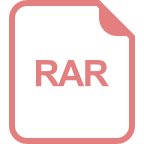
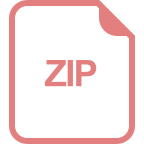
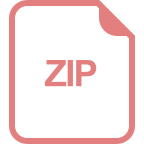
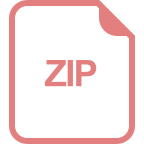
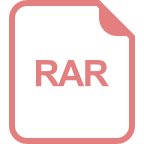