使用got库发送http请求超时重试
时间: 2024-10-24 17:10:12 浏览: 15
Go语言标准库并没有直接提供名为"got"的包,但如果你说的是第三方库"golang.org/x/net/http"里的功能,你可以使用`net/http`包配合一些自定义逻辑来实现HTTP请求的超时重试。下面是一个简单的示例:
```go
package main
import (
"context"
"fmt"
"net/http"
"time"
)
func sendRequest(url string) (*http.Response, error) {
client := &http.Client{
Timeout: time.Second * 5, // 设置默认的超时时间
}
for i := 0; i < 3; i++ { // 这里设置了最多重试三次
ctx, cancel := context.WithTimeout(context.Background(), time.Second*3) // 每次请求增加一点超时时间,如3秒
defer cancel()
resp, err := client.Do(ctx, http.NewRequest(http.MethodGet, url, nil))
if err == nil {
return resp, nil
}
// 如果请求失败,检查是否是网络错误或临时不可达,如果是,则重试
if _, ok := err.(*timeoutError); !ok {
break
}
time.Sleep(time.Second * 2) // 等待一段时间再尝试
}
return nil, fmt.Errorf("Failed to send request after retries: %w", err)
}
type timeoutError interface {
error
http.Error
}
func main() {
url := "https://example.com"
resp, err := sendRequest(url)
if err != nil {
fmt.Println(err)
} else {
defer resp.Body.Close()
fmt.Printf("Response status: %d\n", resp.StatusCode)
}
}
阅读全文
相关推荐
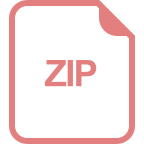
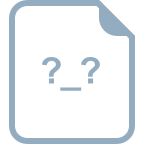

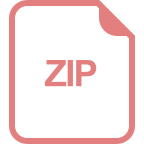
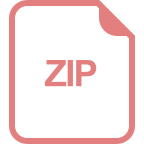
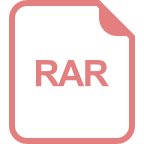
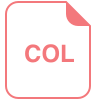
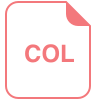
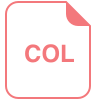
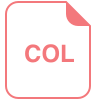
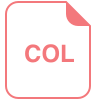
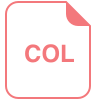
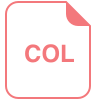
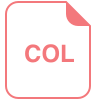
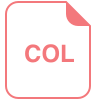
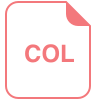
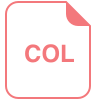
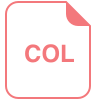
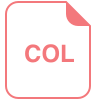