BluetoothGatt 数据据传输 相关代码
时间: 2024-10-19 09:16:21 浏览: 8
Bluetooth GATT(Generic Attribute Profile)是一种蓝牙低功耗(BLE)数据传输协议,用于设备之间的数据交换,尤其是在物联网应用中。在Android中,通过`BluetoothGatt` API可以实现蓝牙设备的数据传输。这里是一个简单的例子,展示如何读取和写入GATT服务和特征值:
```java
// 初始化蓝牙适配器和扫描结果
BluetoothManager bluetoothManager = (BluetoothManager) getSystemService(Context.BLUETOOTH_SERVICE);
BluetoothAdapter adapter = bluetoothManager.getAdapter();
BluetoothDevice device = ...; // 获取已配对的设备
// 连接到蓝牙设备
BluetoothGatt gatt = device.connectGatt(this, false, callback);
// 创建回调处理连接和读写的操作
BluetoothGattCallback callback = new BluetoothGattCallback() {
@Override
public void onConnectionStateChange(BluetoothGatt gatt, int status, int newState) {
if (newState == BluetoothProfile.STATE_CONNECTED) {
// 设备连接成功后,开始发现服务
gatt.discoverServices();
}
}
@Override
public void onServiceDiscovered(BluetoothGatt gatt, List<BluetoothService> services) {
for (BluetoothService service : services) {
// 找到需要的服务,然后获取特征描述符
BluetoothGattCharacteristic characteristic = service.getCharacteristics().get(0); // 假设特征ID是第一个
if (characteristic.getProperties().contains(BluetoothGattCharacteristic.PROPERTY_READ)) {
// 请求读取特性值
gatt.readCharacteristic(characteristic);
}
}
}
@Override
public void onCharacteristicRead(BluetoothGatt gatt, BluetoothGattCharacteristic characteristic, int status) {
if (status == BluetoothGatt.GATT_SUCCESS) {
byte[] value = characteristic.getValue();
// 处理接收到的数据
Log.d("TAG", "Received data: " + Arrays.toString(value));
}
}
@Override
public void onCharacteristicWrite(BluetoothGatt gatt, BluetoothGattCharacteristic characteristic, int status) {
if (status == BluetoothGatt.GATT_SUCCESS) {
// 写入数据完成
Log.d("TAG", "Wrote to characteristic");
}
}
};
// 发送数据示例(假设你想写入特征值)
byte[] writeData = ...; // 需要发送的数据
BluetoothGattCharacteristic writeCharacteristic = ...;
gatt.writeCharacteristic(writeCharacteristic, writeData);
```
阅读全文
相关推荐
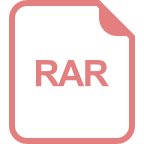
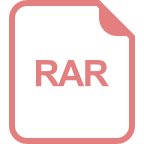
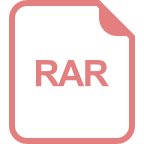

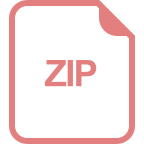
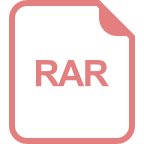
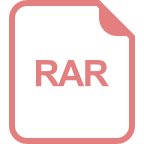
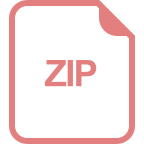
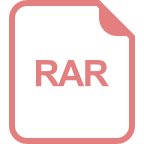
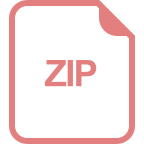
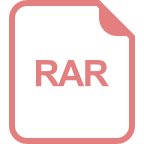
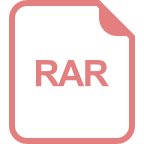
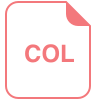
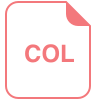
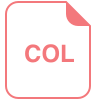



