C++哈希表数据结构
时间: 2023-11-11 08:57:04 浏览: 122
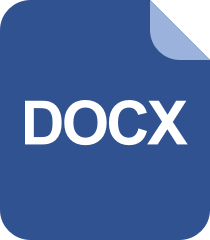
哈希表的数据结构
C中的哈希表是一种使用哈希函数将键映射到存储位置的数据结构。它是一种高效的查找数据结构,可以在平均情况下实现常数时间的插入、删除和查找操作。
在C中,可以使用标准库中的哈希表实现,例如使用unordered_set或unordered_map来创建哈希表。unordered_set用于存储唯一的键,而unordered_map用于存储键值对。
下面是使用C++标准库中的unordered_map实现哈希表的示例代码:
```
#include <unordered_map>
int main() {
// 创建一个哈希表
std::unordered_map<int, std::string> hashMap;
// 向哈希表中插入元素
hashMap.insert({1, "apple"});
hashMap.insert({2, "banana"});
hashMap.insert({3, "orange"});
// 访问哈希表中的元素
std::string fruit = hashMap[1]; // 输出 "apple"
// 修改哈希表中的元素
hashMap[2] = "grape";
// 遍历哈希表中的元素
for (const auto& pair : hashMap) {
int key = pair.first;
std::string value = pair.second;
// 对每个键值对执行操作
}
// 删除哈希表中的元素
hashMap.erase(3);
return 0;
}
```
阅读全文
相关推荐
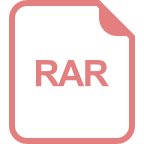
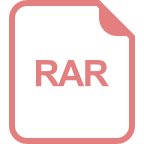
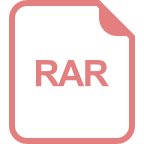
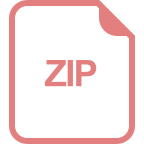
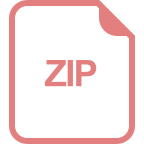
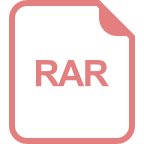
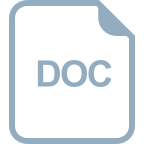
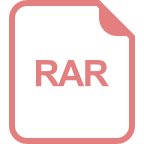
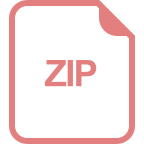
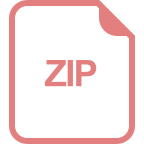
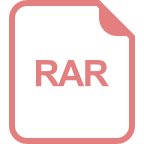
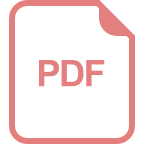