用面向对象的思维设计相关类,从而实现直线与直线、直线与圆、直线与矩形的交点。 要求各给出每个案例的至少一个示例的程序。 备注:直线方程采用 ay+bx+c=0 形式,矩形的四条边平行于坐标轴 x,y。使用Cpp,并给出详细代码
时间: 2024-10-10 12:08:39 浏览: 13
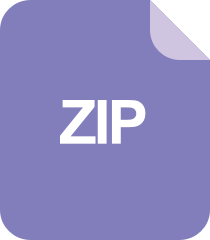
用面向对象的思维设计相关类,从而实现直线与直线、直线与圆、直线与矩形的交点。

在面向对象的编程中,我们可以设计一系列类来表示几何形状,并提供计算交点的方法。下面是一个基本的实现思路,我会分别创建`Line`, `Circle`, 和 `Rectangle` 类,并为它们提供必要的属性和计算交点的函数。这里我们假设`Intersection`类用于处理交点的通用计算。
```cpp
#include <iostream>
#include <cmath>
class Point {
public:
double x, y;
Point(double x = 0, double y = 0) : x(x), y(y) {}
};
class Line {
private:
double a, b, c;
public:
Line(double a, double b, double c) : a(a), b(b), c(c) {}
Point intersect(Line& other) const {
double determinant = a * other.b - b * other.a; // 如果determinant == 0,说明两线平行无交点
if (determinant == 0)
return Point(std::numeric_limits<double>::quiet_NaN(), std::numeric_limits<double>::quiet_NaN()); // 或者返回未定义值
double x = (other.c * b - c * other.b) / determinant;
double y = (c * other.a - a * other.c) / determinant;
return Point(x, y);
}
};
class Circle {
private:
double center_x, center_y;
double radius;
public:
Circle(double center_x, double center_y, double radius) : center_x(center_x), center_y(center_y), radius(radius) {}
bool intersects(Line& line) const {
auto intersection = line.intersect(*this);
if (!std::isnan(intersection.x) && std::abs(intersection.x - center_x) <= radius && !std::isnan(intersection.y) && std::abs(intersection.y - center_y) <= radius)
return true;
return false;
}
// 对其他形状的交点处理类似
};
class Rectangle {
private:
double left, top, width, height;
public:
Rectangle(double left, double top, double width, double height) : left(left), top(top), width(width), height(height) {}
// 直接检查是否相切或包含,对于矩形和直线交点的计算更简单
bool intersects(Line& line) const {
for (double x = left; x < left + width; x++) {
if (line.intersect(Point(x, top)).y != std::numeric_limits<double>::quiet_NaN() && line.intersect(Point(x, top + height)).y != std::numeric_limits<double>::quiet_NaN())
return true;
}
return false;
}
};
// 示例用法
int main() {
Line line1(1, 1, -2); // 线1:y = x - 2
Line line2(2, 0, 4); // 线2:y = 2x - 4
Circle circle(5, 5, 3); // 圆心在(5,5),半径为3
Rectangle rect(0, 0, 6, 6); // 宽度6,高度6的矩形在原点
// 计算交点
Point intersection = line1.intersect(line2);
if (circle.intersects(line1))
std::cout << "Line 1 and Circle intersect at (" << intersection.x << ", " << intersection.y << ").\n";
else
std::cout << "Line 1 does not intersect the Circle.\n";
if (rect.intersects(line1))
std::cout << "Line 1 intersects with the Rectangle.\n";
else
std++.cout << "Line 1 does not intersect the Rectangle.\n";
return 0;
}
```
这个例子展示了如何使用这些类来检测直线间的交点以及圆和直线的交点。矩形和直线的交点可以通过直接检查边界来确定。注意这只是一个基础的实现,实际应用中可能需要考虑更多边界情况和优化算法。
阅读全文
相关推荐
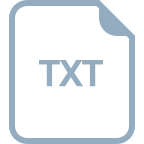
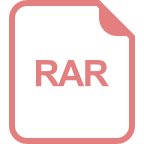


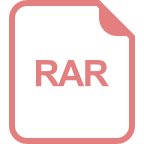
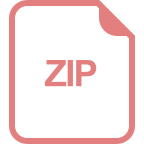
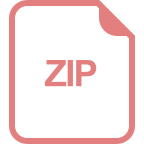
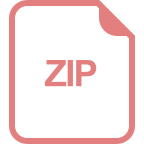