用面向对象的思维设计相关类,从而实现直线与直线、直线与圆、直线与矩形的交点。 要求各给出每个案例的至少一个示例的程序。 备注:直线方程采用 ay+bx+c=0 形式,矩形的四条边平行于坐标轴 x,y。
时间: 2024-10-10 18:02:55 浏览: 32
用面向对象的思维设计此类场景,我们可以创建三个类:`Line`, `Circle`, 和 `Rectangle`,每个类都有其特定的属性(如直线的斜率、截距,圆的半径等)和计算交点的方法。这里提供一些基本的伪代码示例:
```python
# Line 类
class Line:
def __init__(self, a, b, c):
self.a = a
self.b = b
self.c = c
# 计算与其他线段、圆形或矩形的交点
def intersect(self, other):
if isinstance(other, Line):
# 直线与直线相交
intersection = self.solve_line_intersection(other)
elif isinstance(other, Circle):
# 直线与圆相交
intersection = self.solve_circle_intersection(other)
elif isinstance(other, Rectangle):
# 直线与矩形相交
intersection = self.solve_rectangle_intersection(other)
else:
raise TypeError("Unsupported type for intersection")
return intersection
# Circle 类
class Circle:
def __init__(self, center_x, center_y, radius):
self.center = (center_x, center_y)
self.radius = radius
def intersect(self, line):
# 圆心到直线的距离小于等于半径才会有交点
distance = self.distance_from_line(line)
if distance <= self.radius:
# 解出交点,如果可行的话
intersection = self.solve_circle_line_intersection(line)
else:
intersection = None
return intersection
def distance_from_line(self, line):
# 算法略,涉及向量运算
pass
# Rectangle 类
class Rectangle:
def __init__(self, top_left, bottom_right):
self.top_left = top_left
self.bottom_right = bottom_right
def intersect(self, line):
# 检查是否有一条边与直线垂直相交
if line.is_perpendicular_to_axis():
intersection = line.intersect_axis(self)
else:
intersection = None
return intersection
def is_perpendicular_to_axis(self):
# 判断是否与x轴或y轴垂直
pass
# 实现细节函数(例如求解线与线的交点,圆心到线的距离等)
def solve_line_intersection(line1, line2):
pass
def solve_circle_intersection(circle, line):
pass
def solve_rectangle_intersection(rectangle, line):
pass
```
注意这只是一个基础的框架,并未包含所有的计算细节。实际的算法可能涉及到更复杂的几何操作,例如判断两条线是否平行、距离公式以及求解二次方程等。在每个方法中,你需要根据具体的数学原理实现相应的计算。
阅读全文
相关推荐
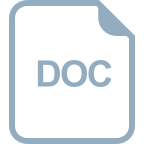
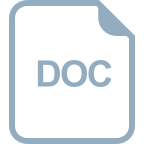
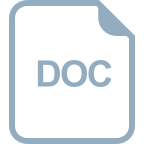
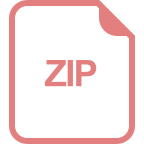

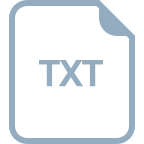
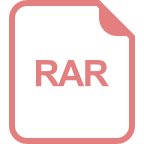
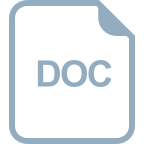
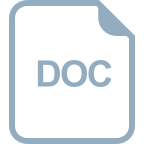
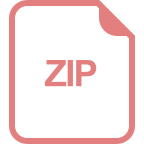
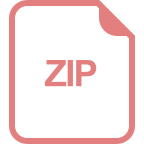
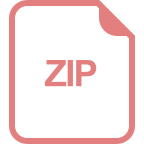
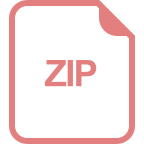
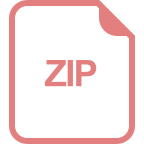
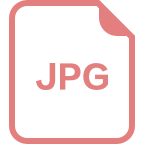
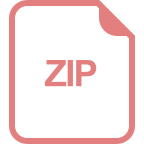