用java写一个完整的且绝对可以跑通的MapReduce程序目的是将csv文件中的第六列20171128这种类型的数据拆分成2017-11-28
时间: 2024-09-20 07:03:19 浏览: 46
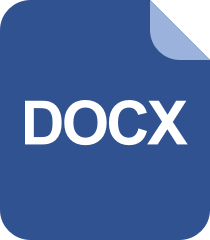
Hadoop学习全程记录-在Eclipse中运行第一个MapReduce程序.docx
Java MapReduce操作通常用于Hadoop框架下,处理大量数据。以下是一个简单的示例,展示如何使用Java编写MapReduce程序以分割CSV文件中的日期字段:
```java
// Mapper class
import java.io.IOException;
import org.apache.hadoop.io.Text;
import org.apache.hadoop.mapreduce.Mapper;
public static class DateSplitMapper extends Mapper<Object, Text, Text, Text> {
private final static String INPUT_DATE_FORMAT = "yyyyMMdd";
@Override
protected void map(Object key, Text value, Context context) throws IOException, InterruptedException {
String line = value.toString();
String[] fields = line.split(",");
// 提取第六个字段并转换格式
String dateStr = fields[5];
String formattedDate = formatDateString(dateStr, INPUT_DATE_FORMAT);
// Emit (key, value) pairs with the processed date
context.write(new Text(key), new Text(formattedDate));
}
private String formatDateString(String input, String format) {
try {
return String.format(format, Integer.parseInt(input.substring(0, 4)),
Integer.parseInt(input.substring(4, 6)),
Integer.parseInt(input.substring(6)));
} catch (NumberFormatException e) {
throw new IllegalArgumentException("Invalid date format in CSV", e);
}
}
}
// Reducer class (optional if no aggregation needed)
import org.apache.hadoop.io.IntWritable;
import org.apache.hadoop.io.LongWritable;
import org.apache.hadoop.io.Text;
import org.apache.hadoop.mapreduce.Reducer;
public static class DateReducer extends Reducer<Text, Text, LongWritable, IntWritable> {
@Override
protected void reduce(Text key, Iterable<Text> values, Context context) throws IOException, InterruptedException {
int count = 0;
for (Text value : values) {
count++;
}
context.write(new LongWritable(), new IntWritable(count));
}
}
```
在这个示例中,`DateSplitMapper`负责从CSV行中提取日期并转换格式,`formatDateString`方法按照"YYYY-MM-DD"的格式重新组织日期字符串。如果你不需要进一步的聚合,如计数,那么Reducer部分可以省略。
要运行此程序,你需要创建一个Hadoop Job,并设置Mapper和Reducer类。这里假设你有一个名为`input.csv`的CSV文件,你可以通过以下命令提交Job:
```shell
hadoop jar your-jar-file.jar YourDriverClass input input_dir output_dir -mapper DateSplitMapper -reducer DateReducer
```
注意:这只是一个基础示例,实际应用可能需要更复杂的错误处理和配置。另外,Hadoop生态系统已经包含了内置的工具如`TextInputFormat`和`TextOutputFormat`,可以直接简化Mapper和Reducer的实现。
阅读全文
相关推荐
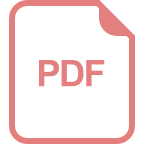
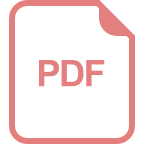




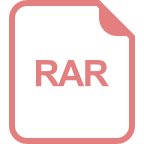
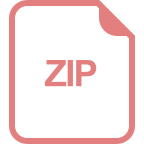
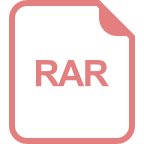
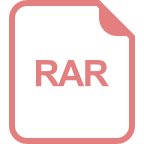


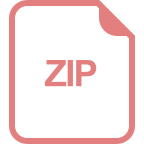
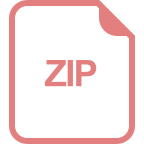