C语言gettimeofday可以和srand一起使用吗
时间: 2024-10-27 21:11:55 浏览: 21
`gettimeofday()` 和 `srand()` 是两个在C语言中用于不同功能的函数。
`gettimeofday()` 函数主要用于获取当前的时间戳,它返回的是一个包含秒数和微秒数的结构体,常用于时间相关的系统调用或精确计时操作。
`srand()` 函数则是初始化随机数生成器的种子值,通常在需要使用随机数生成功能之前调用,以便后续调用 `rand()` 等随机数生成函数能产生一系列看起来随机的整数。
这两个函数的主要作用域和目的并不相同,一个涉及时间处理,另一个涉及随机数生成。它们可以在程序的不同部分并行使用,但在实际应用中并没有直接关联。如果你需要基于当前时间设置随机数的种子,可以先调用 `gettimeofday()` 获取时间戳,然后用其结果作为 `srand()` 的参数:
```c
#include <time.h>
#include <stdlib.h>
// 获取当前时间作为随机数种子
struct timeval now;
gettimeofday(&now, NULL);
unsigned int seed = (now.tv_usec + now.tv_sec) * 1000; // 时间转换为毫秒
// 初始化随机数生成器
srand(seed);
// 使用随机数
int random_num = rand();
```
相关问题
c语言是否可以使用RAND_bytes
C语言标准库中并没有直接提供名为`RAND_bytes`的函数。`RAND_bytes`通常是指某些特定环境下的API,比如OpenSSL库中用于生成随机字节的函数。OpenSSL是一个流行的加密库,在其中你可以找到`RAND_bytes`这样的函数来生成高质量的随机数据。
在纯C语言的标准库中,你可能会使用`rand()`和`srand()`函数来自动生成随机数,但这并不适合生成安全的随机数据,因为它们的种子值可能是固定的,而且生成的随机序列不够随机。
如果你想在C语言中生成高质量的随机字节流,一种常见的做法是使用`arc4random`(对于C99及以上版本)或者第三方库,如cryptopp、mbedTLS等。例如,`arc4random_buf`函数可以从系统熵池获取随机数据。
```c
#include <stdlib.h>
#include <sys/time.h>
size_t get_random_bytes(void *buf, size_t len) {
struct timeval tv;
if (gettimeofday(&tv, NULL) == -1) {
perror("gettimeofday");
exit(EXIT_FAILURE);
}
uint32_t seed = tv.tv_usec + tv.tv_sec;
srand(seed);
return arc4random_buf(buf, len);
}
int main() {
unsigned char bytes[8];
size_t bytes_generated = get_random_bytes(bytes, sizeof(bytes));
assert(bytes_generated == sizeof(bytes)); // 检查生成了预期长度的随机字节
// 然后将bytes作为字符串使用
return 0;
}
```
C语言使用coro实现生产者消费者
使用coroutine实现生产者消费者模型可以很好地展示协程的高效性,下面是一个示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <sys/time.h>
#include "coroutine.h"
#define MAX_SIZE 10
#define PRODUCER_COUNT 3
#define CONSUMER_COUNT 3
// 生产者协程
struct coroutine *producer[MAX_SIZE];
// 消费者协程
struct coroutine *consumer[MAX_SIZE];
// 缓冲区
int buffer[MAX_SIZE];
// 缓冲区的头指针和尾指针
int head = 0;
int tail = 0;
// 生产者线程函数
void producer_func(void *arg)
{
int id = *(int *)arg;
while (1) {
// 判断缓冲区是否已满
while ((tail + 1) % MAX_SIZE == head) {
printf("producer %d: buffer is full, yield...\n", id);
coroutine_yield(producer[id]);
}
// 向缓冲区添加数据
int data = rand() % 100;
buffer[tail] = data;
tail = (tail + 1) % MAX_SIZE;
printf("producer %d: produce data %d, buffer size is %d\n", id, data, (tail - head + MAX_SIZE) % MAX_SIZE);
// 唤醒一个消费者协程
coroutine_resume(consumer[head]);
}
}
// 消费者线程函数
void consumer_func(void *arg)
{
int id = *(int *)arg;
while (1) {
// 判断缓冲区是否为空
while (head == tail) {
printf("consumer %d: buffer is empty, yield...\n", id);
coroutine_yield(consumer[id]);
}
// 从缓冲区取出数据
int data = buffer[head];
head = (head + 1) % MAX_SIZE;
printf("consumer %d: consume data %d, buffer size is %d\n", id, data, (tail - head + MAX_SIZE) % MAX_SIZE);
// 唤醒一个生产者协程
coroutine_resume(producer[id]);
}
}
int main()
{
// 初始化随机数种子
struct timeval tv;
gettimeofday(&tv, NULL);
srand(tv.tv_usec);
// 创建生产者协程
for (int i = 0; i < PRODUCER_COUNT; i++) {
int *id = (int *)malloc(sizeof(int));
*id = i;
producer[i] = coroutine_create(producer_func, id);
}
// 创建消费者协程
for (int i = 0; i < CONSUMER_COUNT; i++) {
int *id = (int *)malloc(sizeof(int));
*id = i;
consumer[i] = coroutine_create(consumer_func, id);
}
// 启动生产者协程
for (int i = 0; i < PRODUCER_COUNT; i++) {
coroutine_resume(producer[i]);
}
// 启动消费者协程
for (int i = 0; i < CONSUMER_COUNT; i++) {
coroutine_resume(consumer[i]);
}
// 等待所有协程执行完毕
while (coroutine_pending()) {
coroutine_schedule();
}
// 销毁协程
for (int i = 0; i < PRODUCER_COUNT; i++) {
coroutine_destroy(producer[i]);
}
for (int i = 0; i < CONSUMER_COUNT; i++) {
coroutine_destroy(consumer[i]);
}
return 0;
}
```
程序中定义了一个大小为10的缓冲区,生产者协程不断向缓冲区添加数据,消费者协程不断从缓冲区取出数据。当缓冲区为空时,消费者协程会yield并等待生产者协程唤醒;当缓冲区已满时,生产者协程会yield并等待消费者协程唤醒。
注意,在生产者和消费者协程中,使用coroutine_yield和coroutine_resume函数实现协程的切换,调度器使用coroutine_schedule函数来进行协程的调度。
阅读全文
相关推荐
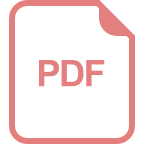
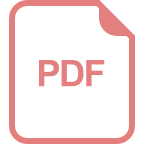
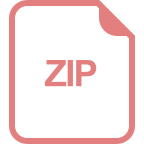
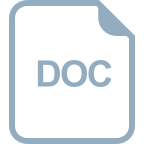
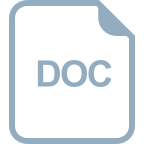
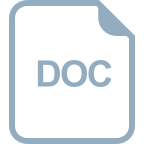
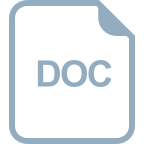
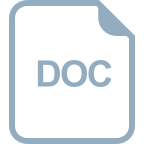





