C++字符串转布尔值
时间: 2024-09-29 14:15:07 浏览: 34
在C++中,将字符串转换为布尔值通常不是直接操作,因为C++标准库本身并没有提供内置的函数将字符串直接映射到布尔类型(true/false)。但是你可以通过编写一些自定义的逻辑来实现这个转换。一种常见的做法是检查字符串是否等于"true"、"false"、"1" 或 "0",然后做相应的转换:
```cpp
#include <string>
#include <sstream>
bool stringToBool(const std::string& str) {
std::istringstream iss(str);
char c;
if (iss >> std::noskipws >> c) {
if (c == 't' || c == 'T') return true; // 转换小写并比较
if (c == 'f' || c == 'F') return false;
if (isdigit(c)) {
int value;
iss >> value;
return value != 0;
}
}
throw std::invalid_argument("Invalid boolean string"); // 非法字符串抛出异常
}
```
相关问题
输入一行字符串表示布尔二叉树的顺序存储,用c++
在C++中,我们可以将布尔二叉树转换成顺序存储,通常称为数组形式,其中每个元素对应于二叉树的一个节点,而元素的值和位置反映了节点的子节点信息。为了实现这一点,我们需要确定一个节点值和它的左、右孩子的关系。一种常见的方法是使用“1”表示有左子节点,“0”表示无,对于右子节点则取反。
例如,给定字符串"10101",可以理解为:
- 第一个“1”代表根节点有左子节点;
- 第二个“0”表示根节点无右子节点;
- 第三个“1”代表左子节点有左子节点;
- 第四个“0”表示左子节点无右子节点;
- 第五个“1”代表左子节点有右子节点。
我们可以这样实现:
```cpp
#include <vector>
#include <string>
class BooleanBinaryTreeNode {
public:
bool data;
BooleanBinaryTreeNode* left;
BooleanBinaryTreeNode* right;
BooleanBinaryTreeNode(bool data) : data(data), left(nullptr), right(nullptr) {}
};
// 根据顺序存储字符串构造二叉树
BooleanBinaryTreeNode* constructBinaryTree(const string& storage) {
int index = 0;
stack<BooleanBinaryTreeNode*> nodes;
nodes.push({storage[index++] - '0'}); // 将字符转为bool
while (!nodes.empty()) {
BooleanBinaryTreeNode* node = nodes.top();
nodes.pop();
// 设置当前节点的左右子节点
if (node->data) {
if (index < storage.length()) {
node->left = new BooleanBinaryTreeNode(storage[index++] - '0');
nodes.push(node->left);
}
if (index < storage.length()) {
node->right = new BooleanBinaryTreeNode(1 - (storage[index++] - '0')); // 取反
nodes.push(node->right);
}
}
}
return nodes.top(); // 返回根节点
}
int main() {
string binaryString = "10101";
BooleanBinaryTreeNode* treeRoot = constructBinaryTree(binaryString);
// ... 使用treeRoot处理和访问二叉树
return 0;
}
```
请注意,这是一个简化的示例,它假设输入字符串总是有效的。实际应用中,你需要添加错误检查和边界条件处理。
c++字符串查看是否重复
在C++中,检查字符串中是否有重复字符可以使用多种方法。以下是一种常见的方法,使用一个标准库中的数据结构和算法来实现:
1. 使用`std::unordered_set`:这个集合不存储重复元素,并且提供了快速的查找功能。通过遍历字符串中的每个字符,并尝试将它们插入到`unordered_set`中。如果插入失败(即返回`false`),则表示字符已经存在,说明字符串中有重复字符。
下面是实现这个方法的示例代码:
```cpp
#include <iostream>
#include <string>
#include <unordered_set>
bool hasDuplicateChars(const std::string& str) {
std::unordered_set<char> chars;
for (char c : str) {
if (chars.find(c) != chars.end()) {
return true; // 字符重复
}
chars.insert(c);
}
return false; // 没有重复字符
}
int main() {
std::string input = "example";
if (hasDuplicateChars(input)) {
std::cout << "字符串中存在重复字符" << std::endl;
} else {
std::cout << "字符串中不存在重复字符" << std::endl;
}
return 0;
}
```
这段代码定义了一个函数`hasDuplicateChars`,它接收一个字符串参数,并返回一个布尔值,指示字符串中是否存在重复字符。在主函数`main`中,它使用一个示例字符串来调用该函数,并打印出相应的结果。
阅读全文
相关推荐
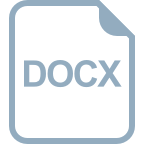
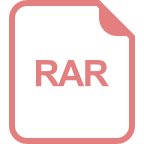
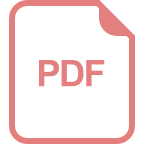
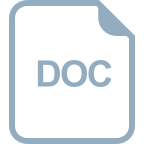
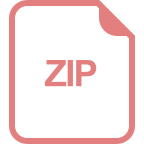
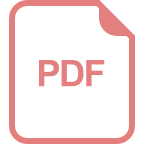
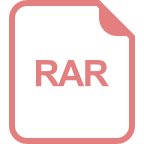
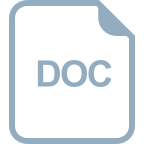
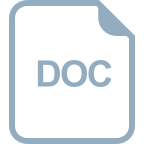
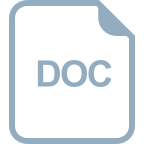
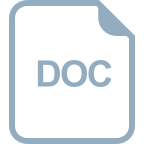
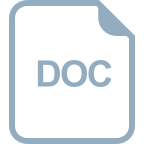
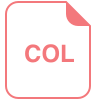
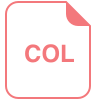



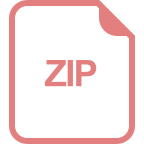