C++判断字符串和另一个字符串是否相等
时间: 2024-09-29 22:11:27 浏览: 45
在C++中,判断两个字符串是否相等通常使用内置的`==`运算符或者成员函数`compare()`。下面是两种常用方法:
1. **使用`==`运算符**:
```cpp
#include <string>
using namespace std;
bool areStringsEqual(string str1, string str2) {
return str1 == str2;
}
```
这里,`str1 == str2`会返回一个布尔值,如果两个字符串内容完全一致则返回`true`,否则`false`。
2. **使用`compare()`函数**:
```cpp
#include <string>
using namespace std;
bool areStringsEqual(string str1, string str2) {
return str1.compare(str2) == 0;
}
```
`compare()`函数会比较两个字符串,如果它们完全相同,`compare()`将返回0。非零值表示两个字符串不同。
注意,在C++中区分大小写,如果需要忽略大小写比较,可以先转换所有字符到小写或大写再进行比较:
```cpp
bool areStringsEqualIgnoreCase(string str1, string str2) {
return tolower(str1) == tolower(str2);
}
```
相关问题
C++判断字符串是否相等
可以使用C++中的字符串比较函数`strcmp()`来判断两个字符串是否相等。如果返回值为0,则表示两个字符串相等,否则不相等。
示例代码:
```c++
#include <iostream>
#include <cstring>
using namespace std;
int main() {
string str1 = "hello";
string str2 = "world";
string str3 = "hello";
if (strcmp(str1.c_str(), str2.c_str()) == 0) {
cout << "str1 and str2 are equal" << endl;
} else {
cout << "str1 and str2 are not equal" << endl;
}
if (strcmp(str1.c_str(), str3.c_str()) == 0) {
cout << "str1 and str3 are equal" << endl;
} else {
cout << "str1 and str3 are not equal" << endl;
}
return 0;
}
```
输出结果:
```
str1 and str2 are not equal
str1 and str3 are equal
```
c++判断字符串是否相等
在C语言中,要判断两个字符串是否相等,可以使用strcmp函数进行比较。strcmp函数会逐个比较两个字符串的字符,直到出现不同的字符或遇到字符串结束符'\0'。如果两个字符串相等,strcmp函数返回0;如果不相等,返回非0值。
示例代码如下:
```c
#include <stdio.h>
#include <string.h>
int main() {
char str1[] = "hello";
char str2[] = "world";
if (strcmp(str1, str2) == 0) {
printf("两个字符串相等\n");
} else {
printf("两个字符串不相等\n");
}
return 0;
}
```
输出结果为:"两个字符串不相等"
阅读全文
相关推荐
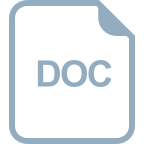
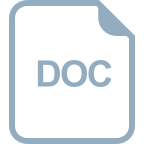
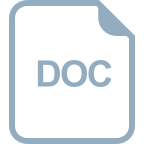













