C++如何判断字符串
时间: 2024-09-13 16:08:04 浏览: 51
在C++中,判断字符串可以有多种方式,具体取决于你想比较的是字符串的值还是地址。以下是几种常见的方法:
1. 使用 `strcmp` 函数比较字符串值:
`strcmp` 函数是C语言标准库中的一个函数,用于比较两个C风格的字符串。如果两个字符串完全相等,`strcmp` 返回0。例如:
```cpp
const char *str1 = "Hello";
const char *str2 = "World";
if (strcmp(str1, str2) == 0) {
// 字符串相等
} else {
// 字符串不相等
}
```
2. 使用C++标准库中的 `std::string` 类的 `==` 操作符:
如果你使用的是C++的 `std::string` 类型的字符串,可以直接使用 `==` 操作符来比较两个字符串是否相等。例如:
```cpp
#include <string>
std::string str1 = "Hello";
std::string str2 = "World";
if (str1 == str2) {
// 字符串相等
} else {
// 字符串不相等
}
```
3. 使用 `std::string` 的 `compare` 方法:
`std::string` 类提供了 `compare` 方法来进行字符串的比较。`compare` 方法在字符串相等时返回0。例如:
```cpp
std::string str1 = "Hello";
std::string str2 = "World";
if (str1.compare(str2) == 0) {
// 字符串相等
} else {
// 字符串不相等
}
```
4. 使用指针比较地址:
如果你想知道两个字符串变量是否指向同一个地址,可以使用 `==` 运算符来比较它们的内存地址。例如:
```cpp
const char *str1 = "Hello";
const char *str2 = "Hello";
const char *str3 = "World";
if (str1 == str2) {
// str1 和 str2 指向同一个地址
} else {
// str1 和 str2 不指向同一个地址
}
if (str1 == str3) {
// str1 和 str3 不指向同一个地址
}
```
需要注意的是,使用指针地址比较的时候,即使两个字符串的内容完全相同,只要它们不是指向同一个内存地址,比较的结果也会是不相等。
阅读全文
相关推荐







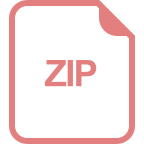








