首页TCPIP_ADAPTER_IF_AP
TCPIP_ADAPTER_IF_AP
时间: 2023-12-13 08:04:38 浏览: 39
TCPIP_ADAPTER_IF_AP是一个枚举类型,表示TCP/IP适配器的接口类型,其中AP表示接入点,即无线接入点。在ESP32中,可以使用TCP/IP适配器API来配置和管理网络接口。例如,可以使用tcpip_adapter_init()函数初始化TCP/IP协议栈,然后使用tcpip_adapter_dhcps_stop()和tcpip_adapter_set_ip_info()函数设置IP地址和网关等信息,最后使用tcpip_adapter_dhcps_start()函数启动DHCP服务器。在这些函数中,需要指定TCP/IP适配器的接口类型,例如TCPIP_ADAPTER_IF_AP表示无线接入点。
CSDN会员
开通CSDN年卡参与万元壕礼抽奖
最新推荐
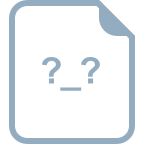
什么是yolov10,简单举例.md
YOLOv10是一种目标检测算法,是YOLO系列算法的第10个版本。YOLO(You Only Look Once)是一种快速的实时目标检测算法,能够在一张图像中同时检测出多个目标。
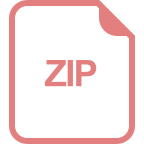
shufflenet模型-图像分类算法对动态表情分类识别-不含数据集图片-含逐行注释和说明文档.zip
shufflenet模型_图像分类算法对动态表情分类识别-不含数据集图片-含逐行注释和说明文档
本代码是基于python pytorch环境安装的。
下载本代码后,有个环境安装的requirement.txt文本
如果有环境安装不会的,可自行网上搜索如何安装python和pytorch,这些环境安装都是有很多教程的,简单的
环境需要自行安装,推荐安装anaconda然后再里面推荐安装python3.7或3.8的版本,pytorch推荐安装1.7.1或1.8.1版本
首先是代码的整体介绍
总共是3个py文件,十分的简便
且代码里面的每一行都是含有中文注释的,小白也能看懂代码
然后是关于数据集的介绍。
本代码是不含数据集图片的,下载本代码后需要自行搜集图片放到对应的文件夹下即可
在数据集文件夹下是我们的各个类别,这个类别不是固定的,可自行创建文件夹增加分类数据集
需要我们往每个文件夹下搜集来图片放到对应文件夹下,每个对应的文件夹里面也有一张提示图,提示图片放的位置
然后我们需要将搜集来的图片,直接放到对应的文件夹下,就可以对代码进行训练了。
运行01生成txt.py,
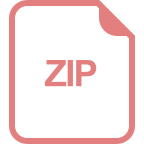
该项目存放基于Cesium的三维GIS平台开发中各种实践程序、截图、总结等,其中程序目录结构
"GIS" 通常指的是 地理信息系统(Geographic Information System)。它是一种特定的空间信息系统,用于捕获、存储、管理、分析、查询和显示与地理空间相关的数据。GIS 是一种多学科交叉的产物,涉及地理学、地图学、遥感技术、计算机科学等多个领域。
GIS 的主要特点和功能包括:
空间数据管理:GIS 能够存储和管理地理空间数据,这些数据可以是点、线、面等矢量数据,也可以是栅格数据(如卫星图像或航空照片)。
空间分析:GIS 提供了一系列的空间分析工具,用于查询、量测、叠加分析、缓冲区分析、网络分析等。
可视化:GIS 能够将地理空间数据以地图、图表等形式展示出来,帮助用户更直观地理解和分析数据。
数据输入与输出:GIS 支持多种数据格式的输入和输出,包括数字线划图(DLG)、数字高程模型(DEM)、数字栅格图(DRG)等。
决策支持:GIS 可以为城市规划、环境监测、灾害管理、交通规划等领域提供决策支持。
随着技术的发展,GIS 已经广泛应用于各个领域,成为现代社会不可或缺的一部分。同时,GIS 也在不断地发展和完善,以适应更多领域的需求。
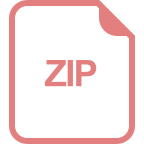
mobilenet模型-基于图像分类算法对猕猴桃品质识别-不含数据集图片-含逐行注释和说明文档.zip
mobilenet模型_基于图像分类算法对猕猴桃品质识别-不含数据集图片-含逐行注释和说明文档
本代码是基于python pytorch环境安装的。
下载本代码后,有个环境安装的requirement.txt文本
如果有环境安装不会的,可自行网上搜索如何安装python和pytorch,这些环境安装都是有很多教程的,简单的
环境需要自行安装,推荐安装anaconda然后再里面推荐安装python3.7或3.8的版本,pytorch推荐安装1.7.1或1.8.1版本
首先是代码的整体介绍
总共是3个py文件,十分的简便
且代码里面的每一行都是含有中文注释的,小白也能看懂代码
然后是关于数据集的介绍。
本代码是不含数据集图片的,下载本代码后需要自行搜集图片放到对应的文件夹下即可
在数据集文件夹下是我们的各个类别,这个类别不是固定的,可自行创建文件夹增加分类数据集
需要我们往每个文件夹下搜集来图片放到对应文件夹下,每个对应的文件夹里面也有一张提示图,提示图片放的位置
然后我们需要将搜集来的图片,直接放到对应的文件夹下,就可以对代码进行训练了。
运行01生成txt.py,
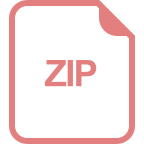
基于Postgres的Dockerfile,包含Postgis GIS扩展、Citus 集群扩展,可用于构建docker镜像
"GIS" 通常指的是 地理信息系统(Geographic Information System)。它是一种特定的空间信息系统,用于捕获、存储、管理、分析、查询和显示与地理空间相关的数据。GIS 是一种多学科交叉的产物,涉及地理学、地图学、遥感技术、计算机科学等多个领域。
GIS 的主要特点和功能包括:
空间数据管理:GIS 能够存储和管理地理空间数据,这些数据可以是点、线、面等矢量数据,也可以是栅格数据(如卫星图像或航空照片)。
空间分析:GIS 提供了一系列的空间分析工具,用于查询、量测、叠加分析、缓冲区分析、网络分析等。
可视化:GIS 能够将地理空间数据以地图、图表等形式展示出来,帮助用户更直观地理解和分析数据。
数据输入与输出:GIS 支持多种数据格式的输入和输出,包括数字线划图(DLG)、数字高程模型(DEM)、数字栅格图(DRG)等。
决策支持:GIS 可以为城市规划、环境监测、灾害管理、交通规划等领域提供决策支持。
随着技术的发展,GIS 已经广泛应用于各个领域,成为现代社会不可或缺的一部分。同时,GIS 也在不断地发展和完善,以适应更多领域的需求。
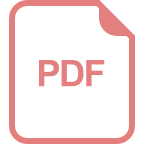
zigbee-cluster-library-specification
最新的zigbee-cluster-library-specification说明文档。

管理建模和仿真的文件
管理Boualem Benatallah引用此版本:布阿利姆·贝纳塔拉。管理建模和仿真。约瑟夫-傅立叶大学-格勒诺布尔第一大学,1996年。法语。NNT:电话:00345357HAL ID:电话:00345357https://theses.hal.science/tel-003453572008年12月9日提交HAL是一个多学科的开放存取档案馆,用于存放和传播科学研究论文,无论它们是否被公开。论文可以来自法国或国外的教学和研究机构,也可以来自公共或私人研究中心。L’archive ouverte pluridisciplinaire
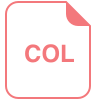
深入了解MATLAB开根号的最新研究和应用:获取开根号领域的最新动态

# 1. MATLAB开根号的理论基础
开根号运算在数学和科学计算中无处不在。在MATLAB中,开根号可以通过多种函数实现,包括`sqrt()`和`nthroot()`。`sqrt()`函数用于计算正实数的平方根,而`nt

react的函数组件的使用
React 的函数组件是一种简单的组件类型,用于定义无状态或者只读组件。 它们通常接受一个 props 对象作为参数并返回一个 React 元素。 函数组件的优点是代码简洁、易于测试和重用,并且它们使 React 应用程序的性能更加出色。 您可以使用函数组件来呈现简单的 UI 组件,例如按钮、菜单、标签或其他部件。 您还可以将它们与 React 中的其他组件类型(如类组件或 Hooks)结合使用,以实现更复杂的 UI 交互和功能。
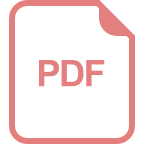
JSBSim Reference Manual
JSBSim参考手册,其中包含JSBSim简介,JSBSim配置文件xml的编写语法,编程手册以及一些应用实例等。其中有部分内容还没有写完,估计有生之年很难看到完整版了,但是内容还是很有参考价值的。