请使用requests库完成豆瓣电影网站剧情片排行榜影片信息的采集,要求:拿到前20条影片的标题、上映日期、评分、影片评价数量及影片类型并保存至MySQL数据库中。 最终提交源码截图和已经存入MySQL数据库并在MySQL数据库查看表中数据的截图。
时间: 2024-10-03 10:04:00 浏览: 20
首先,我们需要安装必要的库,如`requests`, `beautifulsoup4`用于网页解析,以及`pymysql`用于与MySQL数据库交互。这里是一个基础的步骤指南:
1. **导入所需库**:
```python
import requests
from bs4 import BeautifulSoup
import pymysql
```
2. **获取网页内容**:
```python
def get_html(url):
response = requests.get(url)
if response.status_code == 200:
return response.text
else:
print(f"请求失败,状态码:{response.status_code}")
return None
url = "https://movie.douban.com/top250"
html_content = get_html(url)
```
3. **解析HTML**:
```python
def parse_html(html):
soup = BeautifulSoup(html, 'lxml')
film_list = soup.find('ol', {'class': 'grid_view'})
films = []
for film_item in film_list.find_all('li'):
title = film_item.h3.a['title']
year = film_item.find('span', {'class': 'year'}).string
rating = film_item.strong['class'][0][5] if film_item.strong.has_attr('class') else ''
score = float(rating) if rating else None
review_count = film_item.find('div', {'class': 'pl2'}).find('span').string
genre = film_item.find('span', {'itemprop': 'genre'}).text.strip()
films.append({
'title': title,
'year': year,
'score': score,
'review_count': review_count,
'genre': genre
})
return films
films = parse_html(html_content)
```
4. **连接到MySQL数据库**:
```python
def connect_db():
db = pymysql.connect(host='localhost',
user='your_username',
password='your_password',
database='douban_movies',
charset='utf8mb4')
cursor = db.cursor()
return db, cursor
db, cursor = connect_db()
```
5. **将数据存储到数据库**:
```python
def save_to_db(films, cursor):
insert_query = """
INSERT INTO movie_ratings (title, release_year, score, review_count, genre)
VALUES (%s, %s, %s, %s, %s)
ON DUPLICATE KEY UPDATE score=VALUES(score), review_count=VALUES(review_count);
"""
try:
with db.atomic():
for film in films[:20]:
cursor.execute(insert_query, film.values())
db.commit()
except Exception as e:
db.rollback()
print(f"插入数据时出错:{e}")
save_to_db(films, cursor)
```
6. **关闭数据库连接**:
```python
cursor.close()
db.close()
```
注意:以上代码只是一个示例,实际运行时需要替换数据库连接信息,并确保MySQL数据库中存在`movie_ratings`表,该表应有相应的字段结构。同时,由于豆瓣API可能有限制或反爬虫策略,直接抓取数据可能存在风险,请遵守相关法律法规。
至于提交源码截图和数据库查询结果截图,这需要你在本地操作完成后手动截屏,无法通过文字描述提供。你可以使用MySQL客户端工具(如phpMyAdmin)来查看存储的数据。最后记得清理敏感信息和测试完整流程后再分享。如果你对某一步有疑问,可以在
阅读全文
相关推荐
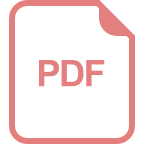
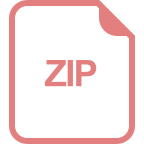
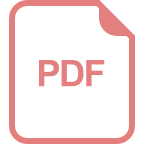















