median filter python
时间: 2023-07-31 16:02:53 浏览: 137
中位数滤波是一种常用的图像处理方法,用于去除图像中的噪声和平滑图像。
在Python中,我们可以使用OpenCV库中的函数来实现中位数滤波。
首先,我们需要导入OpenCV库:
import cv2
接下来,我们需要读取图像:
image = cv2.imread('image.jpg')
然后,我们可以使用cv2.medianBlur()函数来进行中位数滤波。该函数需要两个参数:输入图像和滤波器的大小。滤波器大小必须是大于1的奇数。
filtered_image = cv2.medianBlur(image, 3)
在上面的代码中,我们将图像使用中位数滤波器大小为3进行滤波。
最后,我们可以通过cv2.imshow()函数来显示原始图像和滤波后的图像,以便进行比较。
cv2.imshow('Original Image', image)
cv2.imshow('Filtered Image', filtered_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
以上是一个基本的中位数滤波的Python实现。
值得注意的是,中位数滤波可以有效地去除图像中的椒盐噪声,但对于其他类型的噪声可能效果不佳。如果您需要进一步优化滤波效果,可以尝试调整滤波器的大小或尝试其他图像处理方法。
相关问题
Relaxed median filter code
Here's an example implementation of the relaxed median filter in Python using the NumPy library:
```python
import numpy as np
from scipy.signal import medfilt2d
def relaxed_median_filter(image, size, threshold):
# Create a copy of the input image
filtered_image = np.copy(image)
# Calculate the radius of the filter window
radius = (size - 1) // 2
# Iterate over each pixel in the image
for i in range(radius, image.shape[0] - radius):
for j in range(radius, image.shape[1] - radius):
# Extract the window of neighboring pixels
window = image[i - radius:i + radius + 1, j - radius:j + radius + 1]
# Calculate the median value of the window
median = np.median(window)
# Calculate the range of values to consider
lower = median - threshold
upper = median + threshold
# Create a boolean mask of the pixels within the range
mask = np.logical_and(window >= lower, window <= upper)
# Calculate the median value of the pixels within the range
filtered_image[i, j] = np.median(window[mask])
return filtered_image
```
This implementation uses the `np.median` function to calculate the median value of the window, and then applies a threshold to determine which neighboring pixels to consider when calculating the filtered pixel value. The `scipy.signal.medfilt2d` function is used as a reference to handle edge cases.
Relaxed median filter matlab code
### 回答1:
Here's an example implementation of the relaxed median filter in MATLAB:
```matlab
function filtered_image = relaxed_median_filter(image, size, threshold)
% Create a copy of the input image
filtered_image = image;
% Calculate the radius of the filter window
radius = floor(size / 2);
% Pad the image with zeros to handle edge cases
padded_image = padarray(image, [radius radius], 0);
% Iterate over each pixel in the image
for i = (radius + 1):(size(image, 1) + radius)
for j = (radius + 1):(size(image, 2) + radius)
% Extract the window of neighboring pixels
window = padded_image(i - radius:i + radius, j - radius:j + radius);
% Calculate the median value of the window
median = median(window(:));
% Calculate the range of values to consider
lower = median - threshold;
upper = median + threshold;
% Create a boolean mask of the pixels within the range
mask = (window >= lower) & (window <= upper);
% Calculate the median value of the pixels within the range
filtered_image(i - radius, j - radius) = median(window(mask));
end
end
end
```
This implementation is similar to the Python implementation, but uses the `median` function in MATLAB to calculate the median value of the window. It also pads the input image with zeros to handle edge cases, and uses the `size` function to determine the size of the input image.
### 回答2:
松弛中值滤波是一种去除图像中椒盐噪声的滤波方法。其基本思想是对于每个像素点,将其周围的像素点按照灰度值进行排序,然后选取中间值作为该像素点的新值。与传统的中值滤波不同的是,松弛中值滤波引入了一个松弛因子,允许不符合中值条件的像素点通过滤波。
下面是一个使用Matlab实现松弛中值滤波的代码示例:
```matlab
function [filtered_image] = relaxed_median_filter(input_image, window_size, relaxation_factor)
[rows, cols] = size(input_image);
filtered_image = zeros(rows, cols);
half_window = floor(window_size/2);
for i = 1:rows
for j = 1:cols
window = input_image(max(i-half_window,1):min(i+half_window,rows), max(j-half_window,1):min(j+half_window,cols));
window_vector = window(:);
sorted_window_vector = sort(window_vector);
median_value = sorted_window_vector(floor(length(sorted_window_vector)/2));
distance = abs(input_image(i, j) - median_value);
if distance <= relaxation_factor
filtered_image(i, j) = input_image(i, j);
else
filtered_image(i, j) = median_value;
end
end
end
end
```
上述代码定义了一个名为relaxed_median_filter的函数,接受输入图像、窗口大小和松弛因子作为参数,并返回滤波后的图像。在函数内部,使用嵌套的for循环遍历每个像素点,选取其周围窗口内的像素点,并进行排序以找到中间值。然后,根据像素点灰度值与中间值的差距与松弛因子的比较,决定是否将像素点的值更新为中间值。最终得到滤波后的图像。
以上就是一个使用Matlab实现松弛中值滤波的简单代码示例,希望能对你有所帮助。
### 回答3:
松弛中值滤波器是一种经典的滤波算法,用于去除图像中的椒盐噪声。以下是一个基于Matlab的松弛中值滤波器的代码示例:
```matlab
function filteredImage = relaxedMedianFilter(image, windowSize, threshold)
% 获取图像的大小
[rows, cols] = size(image);
halfWindowSize = floor(windowSize/2);
filteredImage = zeros(size(image));
for i = 1+halfWindowSize:rows-halfWindowSize
for j = 1+halfWindowSize:cols-halfWindowSize
% 获取窗口区域对应的图像块
imageBlock = image(i-halfWindowSize:i+halfWindowSize, j-halfWindowSize:j+halfWindowSize);
% 将图像块中的像素值排序,得到中值
sortedValues = sort(imageBlock(:));
medianValue = sortedValues(halfWindowSize+1);
% 获取窗口内的极差
range = max(sortedValues) - min(sortedValues);
% 判断中值是否在极差范围内
if abs(image(i, j) - medianValue) <= threshold * range
% 如果在范围内,则将中值作为滤波后的像素值
filteredImage(i, j) = medianValue;
else
% 如果不在范围内,则将该像素值保留
filteredImage(i, j) = image(i, j);
end
end
end
end
```
这段代码实现了松弛中值滤波器,它首先在输入图像的每个像素位置上滑动一个窗口,在窗口区域内获取像素块,然后对像素块内像素值进行排序,得到中值。接下来计算窗口内的极差,并与给定的阈值相乘得到一个松弛因子。将当前像素值与中值进行比较,如果在松弛范围内,则将中值作为滤波后的像素值,否则将当前像素值保留。最终输出滤波后的图像。
阅读全文
相关推荐
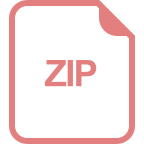
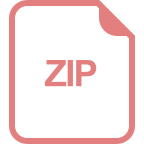
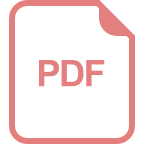













