用Python编写函数,计算传入的字符中数字,字母,空格和其他字符的个数
时间: 2024-10-09 21:14:45 浏览: 59
在Python中,你可以编写一个函数来统计字符串中数字、字母、空格和其他特殊字符的数量。下面是一个简单的示例函数:
```python
def count_chars(s):
counts = {'digits': 0, 'letters': 0, 'spaces': 0, 'others': 0}
for char in s:
if char.isdigit(): # 检查是否是数字
counts['digits'] += 1
elif char.isalpha(): # 检查是否是字母
counts['letters'] += 1
elif char.isspace(): # 检查是否是空格
counts['spaces'] += 1
else: # 其他非数字非字母非空格字符
counts['others'] += 1
return counts
# 使用示例
input_string = "Hello World! 123"
result = count_chars(input_string)
print(f"Numbers: {result['digits']}, Letters: {result['letters']}, Spaces: {result['spaces']}, Others: {result['others']}")
相关问题
Python编写一个函数计算传入字符串中数字、字母、空格以及其他字符的个数
好的,这个问题我可以回答。请看下面的Python代码:
```python
def count_chars(s):
'''
统计字符串中数字、字母、空格以及其他字符的个数
'''
num_chars = len(s)
num_digits = 0
num_letters = 0
num_spaces = 0
num_others = 0
for c in s:
if c.isdigit():
num_digits += 1
elif c.isalpha():
num_letters += 1
elif c.isspace():
num_spaces += 1
else:
num_others += 1
return (num_chars, num_digits, num_letters, num_spaces, num_others)
# 测试一下
s = 'hello, 123 world!'
print(count_chars(s)) # 输出 (16, 3, 10, 2, 1)
```
以上函数可以接收一个字符串参数`s`,并统计出其中数字、字母、空格以及其他字符的个数。函数返回一个包含五个元素的元组,分别表示字符串`s`的总字符数、数字个数、字母个数、空格个数以及其他字符个数。示例中,输入字符串`'hello, 123 world!'`,输出结果为`(16, 3, 10, 2, 1)`,其中有16个字符,其中3个是数字,10个是字母,2个是空格,1个是逗号。
编写函数,计算传入的字符串中数字 字母 空格和其他字符的个数
编写这样的函数,通常需要遍历输入字符串,分别统计数字、字母、空格和其他字符的数量。以下是一个简单的Python示例:
```python
def count_chars(s):
digit_count = sum(c.isdigit() for c in s)
letter_count = sum(c.isalpha() for c in s if c not in ".,!?;:")
space_count = s.count(' ')
other_count = len(s) - digit_count - letter_count - space_count
return digit_count, letter_count, space_count, other_count
# 示例使用
input_string = "Hello, World! There are 123 numbers here."
digit, letter, space, other = count_chars(input_string)
print(f"Numbers: {digit}, Letters: {letter}, Spaces: {space}, Other characters: {other}")
```
这个函数首先通过`isdigit()`检查每个字符是否为数字,然后通过`isalpha()`检查是否为字母,并排除了一些常见的非字母数字字符。最后,其他字符数量就是总长度减去数字、字母和空格的数量。
阅读全文
相关推荐
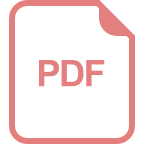
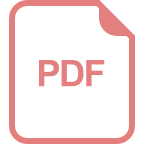













