用python编写出 计算传入的字符串中数字和空格的个数
时间: 2024-04-30 15:24:03 浏览: 103
可以使用Python的字符串方法来实现:
```python
def count_digits_and_spaces(text):
num_digits = sum(c.isdigit() for c in text)
num_spaces = sum(c.isspace() for c in text)
return num_digits, num_spaces
```
通过调用 `isdigit()` 和 `isspace()` 方法,可以分别统计字符串中的数字和空格个数,并返回一个元组。需要注意的是,这个函数只会计算传入字符串中的数字和空格数量,不会计算其他字符的数量。
相关问题
设计一个python函数,计算传入字符串中数字、字母、空格以及其他字符的个数。
当然,我们可以创建一个名为`count_elements`的函数,利用Python内置的`isalnum()`(检查字符是否是字母或数字)、`isspace()`(检查字符是否为空格)和其他条件来统计字符串元素。下面是实现这个功能的代码:
```python
def count_elements(input_string):
digit_count = sum(c.isdigit() for c in input_string)
letter_count = sum(c.isalpha() for c in input_string)
space_count = sum(c.isspace() for c in input_string)
other_count = len(input_string) - digit_count - letter_count - space_count
return digit_count, letter_count, space_count, other_count
# 示例
input_str = "Hello World! 123"
digits, letters, spaces, others = count_elements(input_str)
print(f"Digits: {digits}, Letters: {letters}, Spaces: {spaces}, Other Characters: {others}")
Python编写一个函数计算传入字符串中数字、字母、空格以及其他字符的个数
好的,这个问题我可以回答。请看下面的Python代码:
```python
def count_chars(s):
'''
统计字符串中数字、字母、空格以及其他字符的个数
'''
num_chars = len(s)
num_digits = 0
num_letters = 0
num_spaces = 0
num_others = 0
for c in s:
if c.isdigit():
num_digits += 1
elif c.isalpha():
num_letters += 1
elif c.isspace():
num_spaces += 1
else:
num_others += 1
return (num_chars, num_digits, num_letters, num_spaces, num_others)
# 测试一下
s = 'hello, 123 world!'
print(count_chars(s)) # 输出 (16, 3, 10, 2, 1)
```
以上函数可以接收一个字符串参数`s`,并统计出其中数字、字母、空格以及其他字符的个数。函数返回一个包含五个元素的元组,分别表示字符串`s`的总字符数、数字个数、字母个数、空格个数以及其他字符个数。示例中,输入字符串`'hello, 123 world!'`,输出结果为`(16, 3, 10, 2, 1)`,其中有16个字符,其中3个是数字,10个是字母,2个是空格,1个是逗号。
阅读全文
相关推荐
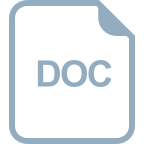
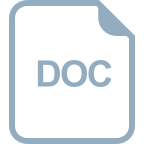
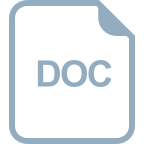













