实现Linux系统从摄像头设备拉流,每5秒拉取1帧图片形成延时摄影文件
时间: 2024-04-14 08:25:42 浏览: 203
要实现Linux系统从摄像头设备拉流,并每5秒拉取1帧图片形成延时摄影文件,你可以使用一些开源的库,如OpenCV和v4l2。以下是一个基本的示例,使用C++和OpenCV库来实现:
1. 安装OpenCV和v4l2库:首先,确保你已经安装了OpenCV和v4l2库。你可以从官方网站下载适合你操作系统的版本,并按照说明进行安装。
2. 编写C++代码:创建一个C++源文件,并使用以下代码来实现从摄像头设备拉流并生成延时摄影文件:
```cpp
#include <opencv2/opencv.hpp>
#include <linux/videodev2.h>
#include <fstream>
int main() {
cv::VideoCapture capture; // 创建视频捕获对象
capture.open("/dev/video0", cv::CAP_V4L2); // 打开摄像头设备
if (!capture.isOpened()) { // 检查设备是否成功打开
std::cerr << "Failed to open camera device!" << std::endl;
return -1;
}
cv::Mat frame; // 存储每一帧图像的变量
int frameCount = 0; // 帧计数器
while (true) {
capture >> frame; // 从摄像头设备读取一帧图像
if (frame.empty()) { // 检查是否读取到了有效的图像
std::cerr << "Failed to read frame!" << std::endl;
break;
}
if (frameCount % 150 == 0) { // 每5秒(150帧)保存一帧图像
std::string filename = "frame_" + std::to_string(frameCount) + ".jpg";
cv::imwrite(filename, frame); // 保存图像为JPEG文件
}
frameCount++; // 帧计数器增加
char key = cv::waitKey(1); // 等待按键输入
if (key == 27) { // 如果按下了Esc键,退出循环
break;
}
}
capture.release(); // 释放摄像头设备资源
return 0;
}
```
3. 编译和运行:使用C++编译器(如g++)编译源文件,并链接OpenCV和v4l2库。
```bash
g++ your_source_file.cpp -o capture_frames `pkg-config --cflags --libs opencv`
```
然后运行可执行文件:
```bash
./capture_frames
```
这样,程序将从摄像头设备拉流,并每5秒保存一帧图像为延时摄影文件。每个图像文件的名称将按照帧计数器进行命名。
请注意,上述代码仅提供了基本的功能实现,你可能需要根据具体需求进行更复杂的图像处理或文件存储操作。此外,确保你有适当的权限来访问摄像头设备(例如,在Linux系统中,通常需要以root用户或有相应权限的用户运行)。
阅读全文
相关推荐

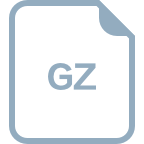
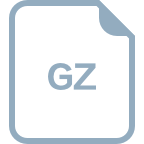











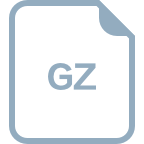


