用 c语言代码建立一个可运行的单链表
时间: 2024-09-23 22:15:37 浏览: 24
在C语言中,我们可以使用结构体和指针来建立一个可运行的单链表。这里是一个简单的示例,展示如何创建节点、初始化链表以及添加节点:
首先,我们定义一个表示节点的结构体:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
struct Node {
int data; // 节点数据
struct Node* next; // 指向下一个节点的指针
};
```
接下来,我们需要创建函数来管理链表:
```c
// 创建一个新的链表节点
struct Node* newNode(int data) {
struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
if (newNode != NULL) {
newNode->data = data;
newNode->next = NULL;
}
return newNode;
}
// 初始化一个空链表
struct Node* createList() {
return NULL;
}
// 添加新节点到链表尾部
void appendNode(struct Node** head, int data) {
struct Node* newNode = newNode(data);
if (*head == NULL) { // 列表为空,将新节点作为头节点
*head = newNode;
} else {
struct Node* current = *head;
while (current->next != NULL) {
current = current->next;
}
current->next = newNode;
}
}
// 打印链表
void printList(struct Node* head) {
while (head != NULL) {
printf("%d -> ", head->data);
head = head->next;
}
printf("NULL\n");
}
```
现在你可以用下面的方式使用这个链表:
```c
int main() {
// 创建链表
struct Node* head = createList();
// 添加节点
appendNode(&head, 1);
appendNode(&head, 2);
appendNode(&head, 3);
// 打印链表
printList(head);
return 0;
}
```
这将创建一个包含数字1, 2, 3的链表,并打印出来。
相关推荐
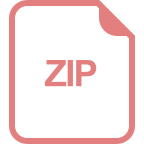
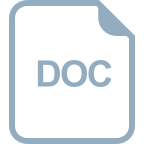















