在VS2010环境下用C语言实现建立单向链表,表长任意;可交互输出单链表中的内容;编写算法计算出自己所建立的单链表的长度并输出;删除自己所建单链表中的第K个节点,并将剩余节点输出;将单链表倒排,输出结果。
时间: 2024-05-08 16:17:47 浏览: 79
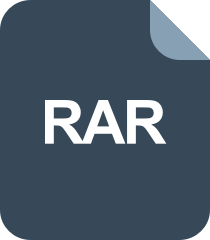
C语言实现单向链表的创建、插入,删除节点,和2个链表合并

以下是实现代码:
```c
#include <stdio.h>
#include <stdlib.h>
struct Node {
int data;
struct Node* next;
};
struct Node* createList();
void printList(struct Node* head);
int getLength(struct Node* head);
struct Node* deleteKthNode(struct Node* head, int k);
struct Node* reverseList(struct Node* head);
int main() {
struct Node* head = createList();
printf("原始链表:");
printList(head);
printf("链表长度:%d\n", getLength(head));
int k;
printf("请输入要删除的节点位置:");
scanf("%d", &k);
head = deleteKthNode(head, k);
printf("删除后的链表:");
printList(head);
printf("倒排后的链表:");
head = reverseList(head);
printList(head);
return 0;
}
struct Node* createList() {
int n, i;
printf("请输入链表长度:");
scanf("%d", &n);
struct Node *head, *p, *q;
head = (struct Node*)malloc(sizeof(struct Node));
p = head;
for (i = 0; i < n; i++) {
q = (struct Node*)malloc(sizeof(struct Node));
printf("请输入第%d个节点的值:", i + 1);
scanf("%d", &q->data);
p->next = q;
p = q;
}
p->next = NULL;
return head;
}
void printList(struct Node* head) {
struct Node* p = head->next;
while (p != NULL) {
printf("%d ", p->data);
p = p->next;
}
printf("\n");
}
int getLength(struct Node* head) {
struct Node* p = head->next;
int len = 0;
while (p != NULL) {
len++;
p = p->next;
}
return len;
}
struct Node* deleteKthNode(struct Node* head, int k) {
struct Node* p = head;
int i = 0;
while (p != NULL && i < k - 1) {
p = p->next;
i++;
}
if (p == NULL || p->next == NULL) {
return head;
}
struct Node* q = p->next;
p->next = q->next;
free(q);
return head;
}
struct Node* reverseList(struct Node* head) {
struct Node *p, *q, *r;
p = head->next;
q = NULL;
while (p != NULL) {
r = p->next;
p->next = q;
q = p;
p = r;
}
head->next = q;
return head;
}
```
使用说明:
1. 编译运行代码;
2. 输入链表长度和每个节点的值;
3. 输出原始链表;
4. 输出链表长度;
5. 输入要删除的节点位置;
6. 输出删除后的链表;
7. 输出倒排后的链表。
阅读全文
相关推荐
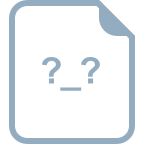

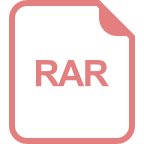
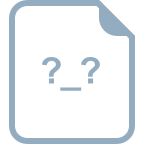
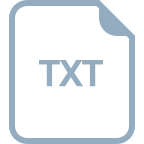
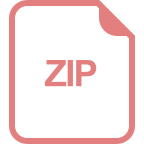
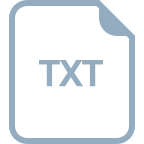
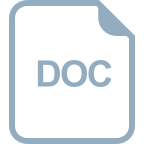
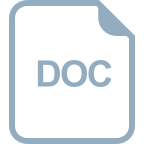
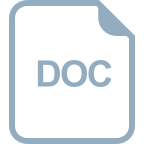
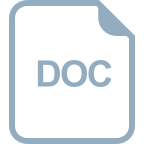
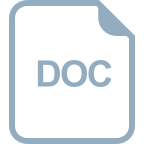
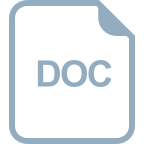
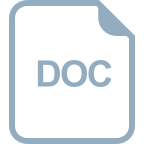
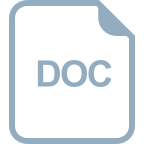
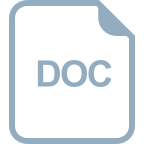
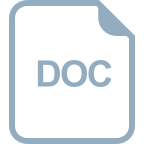