C语言代码实现tar压缩
时间: 2024-04-29 11:17:31 浏览: 272
C语言可以使用系统提供的库函数来实现tar压缩。下面是一个简单的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/stat.h>
#include <fcntl.h>
#include <unistd.h>
#include <dirent.h>
#include <tar.h>
void create_tar(const char* dir_path, const char* tar_path) {
DIR* dir = opendir(dir_path);
if (dir == NULL) {
printf("Failed to open directory.\n");
return;
}
int tar_fd = open(tar_path, O_WRONLY | O_CREAT | O_TRUNC, 0644);
if (tar_fd == -1) {
printf("Failed to create tar file.\n");
closedir(dir);
return;
}
struct dirent* entry;
while ((entry = readdir(dir)) != NULL) {
if (strcmp(entry->d_name, ".") == 0 || strcmp(entry->d_name, "..") == 0) {
continue;
}
char file_path[256];
snprintf(file_path, sizeof(file_path), "%s/%s", dir_path, entry->d_name);
struct stat st;
if (lstat(file_path, &st) == -1) {
printf("Failed to get file status.\n");
continue;
}
struct posix_header header;
memset(&header, 0, sizeof(header));
strncpy(header.name, entry->d_name, sizeof(header.name));
snprintf(header.mode, sizeof(header.mode), "%07o", st.st_mode & 0777);
snprintf(header.uid, sizeof(header.uid), "%07o", st.st_uid);
snprintf(header.gid, sizeof(header.gid), "%07o", st.st_gid);
snprintf(header.size, sizeof(header.size), "%011llo", (unsigned long long)st.st_size);
snprintf(header.mtime, sizeof(header.mtime), "%011lo", (unsigned long)st.st_mtime);
strncpy(header.magic, TMAGIC, sizeof(header.magic));
strncpy(header.version, TVERSION, sizeof(header.version));
if (write(tar_fd, &header, sizeof(header)) == -1) {
printf("Failed to write header.\n");
continue;
}
if (S_ISREG(st.st_mode)) {
int file_fd = open(file_path, O_RDONLY);
if (file_fd == -1) {
printf("Failed to open file.\n");
continue;
}
char buffer[4096];
ssize_t read_bytes;
while ((read_bytes = read(file_fd, buffer, sizeof(buffer))) > 0) {
if (write(tar_fd, buffer, read_bytes) == -1) {
printf("Failed to write file content.\n");
break;
}
}
close(file_fd);
}
}
closedir(dir);
close(tar_fd);
}
int main() {
const char* dir_path = "/path/to/directory";
const char* tar_path = "/path/to/archive.tar";
create_tar(dir_path, tar_path);
return 0;
}
```
上述代码中,`create_tar`函数用于创建tar压缩文件。它首先打开指定的目录,然后遍历目录中的文件和子目录。对于每个文件,它会获取文件的元数据,并将元数据写入tar文件的头部。如果文件是普通文件,则将文件内容写入tar文件。
你可以将`/path/to/directory`替换为你要压缩的目录的路径,将`/path/to/archive.tar`替换为你要生成的tar文件的路径。编译并运行该代码,即可生成tar压缩文件。
阅读全文
相关推荐
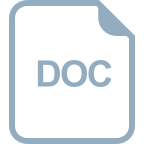
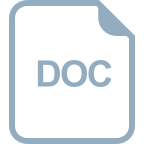
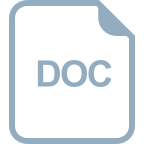
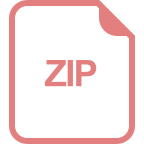
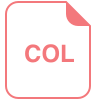
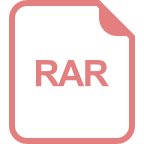
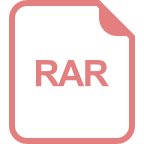
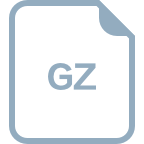
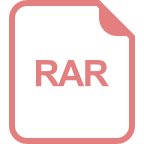
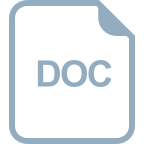
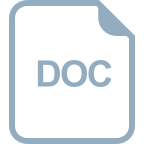
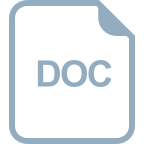
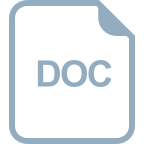
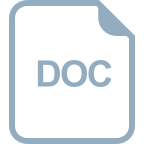
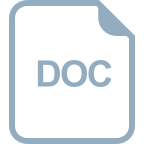
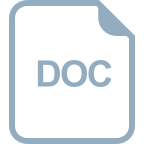
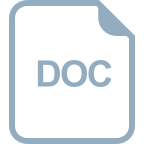

