python在窗体中显示图片
时间: 2024-09-11 10:11:54 浏览: 39
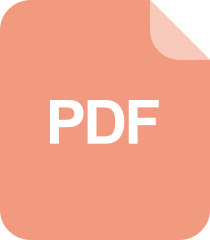
python在新的图片窗口显示图片(图像)的方法
在Python中,特别是在使用GUI库如Tkinter、PyQt、wxPython等创建窗口应用程序时,显示图片通常涉及以下几个步骤:
1. 导入所需的库:如果是Tkinter,你需要`PIL`(Python Imaging Library)或其更现代的替代品`Pillow`来处理图像。
```python
from tkinter import *
from PIL import Image, ImageTk
```
2. 加载图片:使用Image模块的`open()`函数加载图片文件。
```python
image_path = 'path_to_your_image.jpg'
img = Image.open(image_path)
```
3. 调整图片大小(可选):如果需要调整图片尺寸以适应窗体,可以使用`resize()`方法。
```python
img_resized = img.resize((width, height))
```
4. 将图片转换为Tkinter可用的格式:使用`ImageTk.PhotoImage()`将PIL图片对象转换为Tkinter的图片对象。
```python
photo = ImageTk.PhotoImage(img_resized)
```
5. 在窗体上显示图片:创建一个Label对象,并设置其图像属性为刚刚创建的PhotoImage。
```python
canvas = Canvas(root, width=width, height=height)
canvas.create_image(0, 0, anchor=NW, image=photo)
canvas.pack()
```
6. 最后,记得在主循环中运行应用以保持窗口可见。
完整示例:
```python
import tkinter as tk
from PIL import Image, ImageTk
root = tk.Tk()
root.title('显示图片')
# 加载图片并调整大小
image_path = 'path_to_your_image.jpg'
img = Image.open(image_path).resize((400, 400))
# 创建 PhotoImage 对象
photo = ImageTk.PhotoImage(img)
# 创建标签并放置图片
label = tk.Label(root, image=photo)
label.image = photo # 保存引用防止垃圾回收
label.pack()
root.mainloop()
```
阅读全文
相关推荐
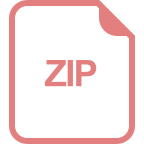
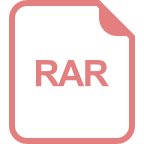


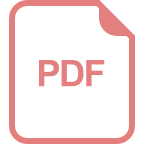
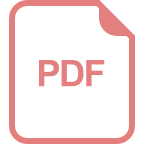
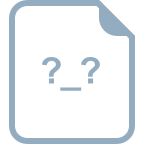
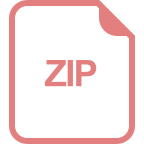
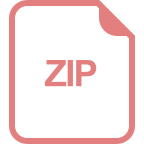
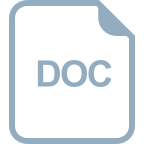
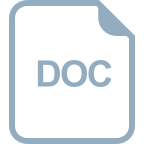
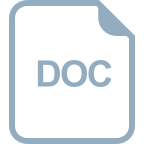




