信道编码汉明码 练习题
时间: 2025-01-07 17:55:51 浏览: 13
### 关于汉明码信道编码的练习题
#### 示例一:计算最小距离并检测错误能力
假设有一个(7, 4)汉明码,其生成矩阵如下所示:
| G = | 1 0 0 0 |
| 0 1 0 0 |
| 0 0 1 0 |
| 0 0 0 1 |
| 1 1 0 1 |
| 1 0 1 1 |
| 0 1 1 1 |
给定消息位`m=[1 0 1 1]`。
为了找到对应的码字c,可以将消息向量乘以生成矩阵G得到结果[^1]:
```matlab
% MATLAB code to calculate codeword from message bits using generator matrix
m = [1 0 1 1]; % Message vector
G = [
1 0 0 0;
0 1 0 0;
0 0 1 0;
0 0 0 1;
1 1 0 1;
1 0 1 1;
0 1 1 1];
c = mod(m * G',2); % Codeword calculation with modulo-2 arithmetic
disp(c);
```
此段代码展示了如何通过MATLAB实现从信息比特到具体码字转换的过程。对于给出的信息序列\[ m \]=\[1\ 0\ 1\ 1\], 计算得出相应的码字为\[ c \]=[1 0 1 1 0 0 0].
接下来考虑传输过程中可能出现单一位错的情况。如果接收到的数据是\[ r \]=[1 0 **0** 1 0 0 0](注意第三位发生了改变),那么可以通过校验方程来判断是否存在错误以及定位错误位置[^2]。
---
#### 示例二:构建奇偶校验矩阵H
对于上述提到的(7, 4)汉明码而言,除了利用生成矩阵外还可以借助奇偶校验矩阵来进行编解码操作。该类码型所使用的标准形式下的检验矩阵通常表示为:
| H = | 1 0 1 0 1 0 1 |
| 0 1 1 0 0 1 1 |
| 0 0 0 1 1 1 1 |
当接收端获得疑似含误码的消息r时,则可通过下述方法验证数据准确性并尝试纠正潜在问题[^3]:
```python
import numpy as np
def syndrome_calculation(received_word, parity_check_matrix):
s = received_word @ parity_check_matrix.T % 2
return ''.join([str(bit) for bit in s])
received_word = np.array([1, 0, 0, 1, 0, 0, 0])
parity_check_matrix = np.array([
[1, 0, 1, 0, 1, 0, 1],
[0, 1, 1, 0, 0, 1, 1],
[0, 0, 0, 1, 1, 1, 1]])
syndrome = syndrome_calculation(received_word, parity_check_matrix)
print(f"The calculated syndrome is {syndrome}.")
if int(syndrome, base=2)==0:
print("No error detected.")
else:
position=int(syndrome[::-1],base=2)+1
corrected_word=list(map(int,reversed(bin(sum((list(reversed(received_word)))*position))[2:].zfill(len(received_word)))))
print(f"Error at position {position}, Corrected word:",corrected_word[-len(received_word):][::-1])
```
这段Python脚本实现了基于奇偶校验矩阵的方法去识别和修复单一比特翻转引起的通信差错的功能。它会先求得综合症值s;若s不等于零则说明确实存在异常情况发生,并据此推断出具体的出错部位以便实施修正措施[^4]。
阅读全文
相关推荐










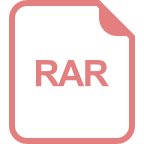
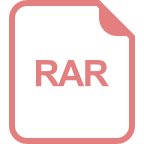
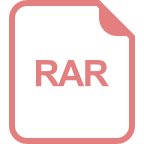
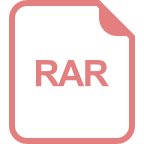
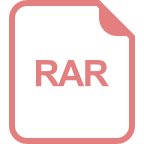
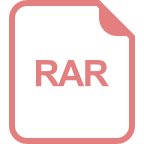
