使用C++完成下面题目:题目:链表的创建、遍历、查找、插入、删除等 (假设链表表元素类型设定为整型) (1)初始化StatusInitList(Linklist&L)////链表表的初始化,构
时间: 2024-09-26 21:05:35 浏览: 29
在C++中,你可以使用结构体和指针来实现链表的基本操作。首先,我们需要定义一个节点结构,包含整数值和指向下一个节点的指针:
```cpp
struct Node {
int data;
Node* next;
};
```
接下来,我们实现链表类,并添加相应的函数:
```cpp
class LinkedList {
public:
// 构造函数和析构函数
LinkedList() : head(nullptr) {}
~LinkedList();
// 初始化链表
void StatusInitList();
// 遍历链表
void Display() const;
// 查找元素
bool Find(int value) const;
// 插入元素
void Insert(int value);
// 删除元素
void Remove(int value);
private:
Node* head; // 指向链表头的指针
};
// 实现函数
void LinkedList::StatusInitList() {
head = nullptr;
}
// 显示链表
void LinkedList::Display() const {
Node* current = head;
while (current != nullptr) {
std::cout << current->data << " ";
current = current->next;
}
std::cout << std::endl;
}
bool LinkedList::Find(int value) const {
Node* current = head;
while (current != nullptr) {
if (current->data == value) return true;
current = current->next;
}
return false;
}
// 插入元素到链表尾部
void LinkedList::Insert(int value) {
Node* newNode = new Node{value, nullptr};
if (head == nullptr) {
head = newNode;
} else {
Node* temp = head;
while (temp->next != nullptr) temp = temp->next;
temp->next = newNode;
}
}
// 删除指定值的元素
void LinkedList::Remove(int value) {
if (head == nullptr) return;
if (head->data == value) {
Node* temp = head;
head = head->next;
delete temp;
return;
}
Node* current = head;
while (current->next != nullptr && current->next->data != value) {
current = current->next;
}
if (current->next != nullptr) {
Node* toDelete = current->next;
current->next = current->next->next;
delete toDelete;
}
}
```
以上就是使用C++实现链表的基本操作的简单示例。记得在实际项目中处理好内存管理,特别是在动态分配和释放节点时。
阅读全文
相关推荐
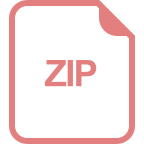
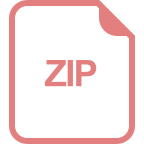
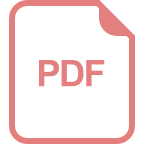
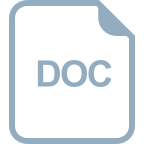
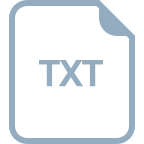
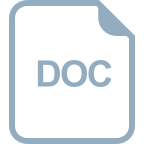
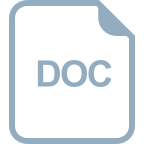
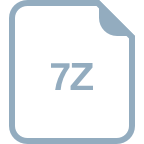
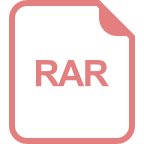
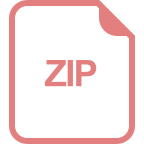
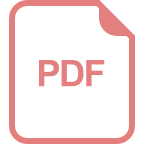
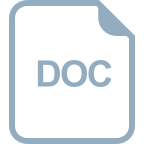
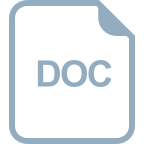
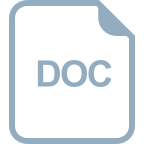
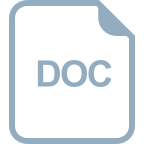
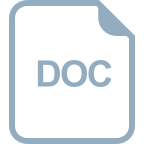
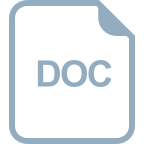