用C语言完成一个链表基本操作编程:初始化、插入、删除、遍历。
时间: 2023-10-24 22:06:59 浏览: 143
好的,以下是用C语言实现链表基本操作的代码:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表结构体
struct Node {
int data;
struct Node* next;
};
// 初始化链表,返回头结点
struct Node* initList() {
struct Node* head = (struct Node*) malloc(sizeof(struct Node));
head->next = NULL;
return head;
}
// 在链表中插入一个结点,data为插入的数据
void insertNode(struct Node* head, int data) {
struct Node* p = (struct Node*) malloc(sizeof(struct Node));
p->data = data;
p->next = head->next;
head->next = p;
}
// 在链表中删除一个结点,data为要删除的数据
void deleteNode(struct Node* head, int data) {
struct Node* p = head->next;
struct Node* q = head;
while (p) {
if (p->data == data) {
q->next = p->next;
free(p);
return;
}
q = p;
p = p->next;
}
}
// 遍历链表,打印所有结点的数据
void traverseList(struct Node* head) {
struct Node* p = head->next;
while (p) {
printf("%d ", p->data);
p = p->next;
}
printf("\n");
}
int main() {
struct Node* head = initList();
insertNode(head, 1);
insertNode(head, 2);
insertNode(head, 3);
printf("链表初始状态:");
traverseList(head);
deleteNode(head, 2);
printf("删除元素2后:");
traverseList(head);
return 0;
}
```
输出结果为:
```
链表初始状态:3 2 1
删除元素2后:3 1
```
以上就是用C语言实现链表基本操作的代码。
阅读全文
相关推荐
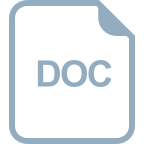
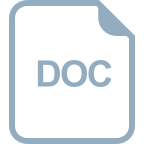
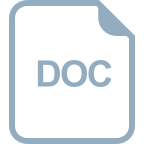
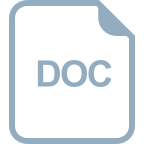
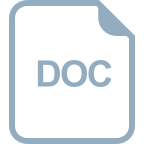
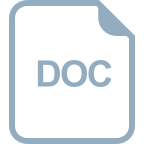
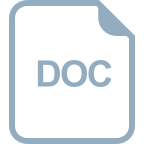
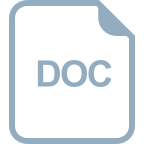
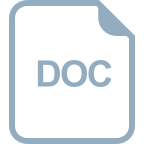
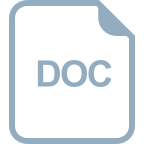
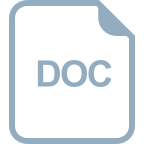
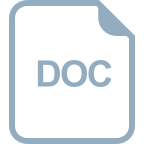
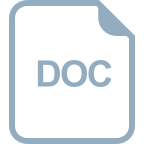
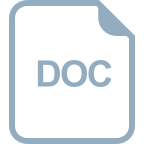
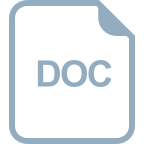
