用c语言写一个不带头结点的循环链表的插入和删除算法及其主函数
时间: 2024-02-06 11:09:42 浏览: 81
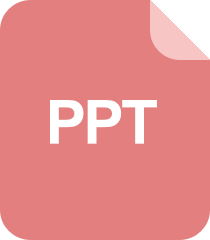
前插入结点算法-关于c语言链表的ppt
以下是不带头结点的循环链表的插入和删除算法及其主函数的C语言代码:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义循环链表结构体
typedef struct node {
int data;
struct node *next;
} Node;
// 循环链表的插入操作
void insert(Node **head, int data, int pos) {
Node *new_node = (Node *)malloc(sizeof(Node));
new_node->data = data;
new_node->next = NULL;
if (*head == NULL) {
// 如果链表为空,则直接将新节点作为头节点
*head = new_node;
new_node->next = *head;
} else if (pos == 1) {
// 如果插入位置为1,则将新节点作为头节点
new_node->next = (*head)->next;
(*head)->next = new_node;
int tmp = (*head)->data;
(*head)->data = new_node->data;
new_node->data = tmp;
} else {
// 如果插入位置不为1,则遍历链表找到插入位置
Node *cur = *head;
int i;
for (i = 1; i < pos - 1 && cur->next != *head; i++) {
cur = cur->next;
}
new_node->next = cur->next;
cur->next = new_node;
}
}
// 循环链表的删除操作
void delete(Node **head, int pos) {
if (*head == NULL) {
// 如果链表为空,则直接返回
return;
} else if (pos == 1) {
// 如果删除位置为1,则将头节点删除
Node *del_node = *head;
if (*head == (*head)->next) {
// 如果链表只有一个节点,则将头节点置为NULL
*head = NULL;
} else {
// 否则将头节点指向下一个节点,并删除原头节点
*head = (*head)->next;
Node *cur = *head;
while (cur->next != del_node) {
cur = cur->next;
}
cur->next = *head;
}
free(del_node);
} else {
// 如果删除位置不为1,则遍历链表找到删除位置
Node *cur = *head;
int i;
for (i = 1; i < pos - 1 && cur->next != *head; i++) {
cur = cur->next;
}
if (cur->next == *head) {
// 如果删除位置超出链表长度,则直接返回
return;
}
Node *del_node = cur->next;
cur->next = del_node->next;
free(del_node);
}
}
// 主函数
int main() {
Node *head = NULL; // 初始化循环链表为空
// 测试插入操作
insert(&head, 1, 1); // 在空链表中插入第一个节点
insert(&head, 2, 1); // 将2插入到头部
insert(&head, 3, 3); // 将3插入到第三个位置
insert(&head, 4, 2); // 将4插入到第二个位置
// 打印链表
printf("循环链表:");
Node *cur = head;
do {
printf("%d ", cur->data);
cur = cur->next;
} while (cur != head);
printf("\n");
// 测试删除操作
delete(&head, 3); // 删除第三个节点
delete(&head, 1); // 删除头节点
delete(&head, 5); // 删除超出链表长度的节点
// 打印链表
printf("循环链表:");
cur = head;
do {
printf("%d ", cur->data);
cur = cur->next;
} while (cur != head);
printf("\n");
return 0;
}
```
在上面的代码中,我们定义了一个循环链表结构体`Node`,其中包含了数据域`data`和指针域`next`。然后,我们实现了循环链表的插入操作`insert`和删除操作`delete`,并在主函数中进行了测试。需要注意的是,在插入操作中,我们需要判断链表是否为空以及插入位置是否为1;在删除操作中,我们需要判断链表是否为空以及删除位置是否超出链表长度。同时,由于是循环链表,我们需要特别处理头节点的删除。
阅读全文
相关推荐
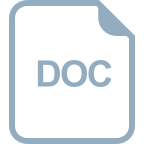
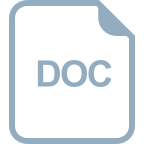



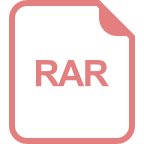











