用C语言编写一个程序,使用指针遍历字符串并计算其中大写字母的数量、小写字母的数量、空格的数量以及数字的数量,请给出相应的代码实现和步骤解释。
时间: 2024-12-24 17:30:31 浏览: 28
在C语言中,我们可以创建一个函数,接受一个字符串作为输入,并使用指针遍历它,同时使用条件语句来区分字符类别。以下是代码实现和步骤说明:
```c
#include <stdio.h>
#include <ctype.h> // 包含ctype.h库,用于检查字符类型
void count_chars(char* str) {
int uppercase = 0;
int lowercase = 0;
int spaces = 0;
int digits = 0;
while (*str != '\0') { // 遍历直到字符串结束
if (isupper(*str)) { // 检查是否为大写字母
uppercase++;
} else if (islower(*str)) { // 检查是否为小写字母
lowercase++;
} else if (*str == ' ') { // 检查是否为空格
spaces++;
} else if (isdigit(*str)) { // 检查是否为数字
digits++;
}
str++; // 移动指针到下一个字符
}
printf("Uppercase letters: %d\n", uppercase);
printf("Lowercase letters: %d\n", lowercase);
printf("Spaces: %d\n", spaces);
printf("Digits: %d\n", digits);
}
int main() {
char str[] = "Hello World! 123";
count_chars(str);
return 0;
}
```
步骤解释:
1. 定义四个整数变量,分别用于存储大写字母、小写字母、空格和数字的数量。
2. 使用`while`循环遍历字符串,直到遇到字符串结束符'\0'。
3. 在循环内部,使用`isupper`、`islower`、`== ' '`和`isdigit`函数检查当前字符的类型,然后相应地增加计数器。
4. 循环结束后,打印出各类字符的数量。
阅读全文
相关推荐
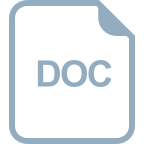
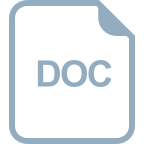
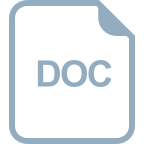















