单向循环链表类c++实现
时间: 2024-09-21 18:07:32 浏览: 61
单向循环链表是一种特殊的链表结构,其中最后一个节点的指针指向第一个节点,形成一个环形。这种数据结构通常用于需要频繁从头开始遍历的情况,比如音乐播放列表或游戏中的队列。下面是一个简单的C++实现:
```cpp
#include <iostream>
// 定义节点结构体
struct Node {
int data;
Node* next; // 指向下一个节点的指针
};
class CircularLinkedList {
private:
Node* head; // 链表头部
public:
// 构造函数,初始化链表为空
CircularLinkedList() : head(nullptr) {}
// 添加新元素到链表尾部并连接形成循环
void addNode(int value) {
if (head == nullptr) {
head = new Node{value, head};
} else {
Node* newNode = new Node{value, head->next};
head->next->next = newNode;
head->next = newNode;
}
}
// 打印链表所有元素(循环遍历)
void display() const {
if (head != nullptr) {
Node* temp = head;
do {
std::cout << temp->data << " ";
temp = temp->next;
} while (temp != head);
}
std::cout << "\n";
}
// 删除指定值的第一个节点(若存在),仅适用于非空链表
bool removeNode(int value) {
if (head == nullptr || head->data != value) return false;
Node* current = head;
while (current->next != head) {
if (current->next->data == value) {
Node* toDelete = current->next;
current->next = current->next->next;
delete toDelete;
return true;
}
current = current->next;
}
return false; // 如果找到的是头结点并且不是目标值,则链表为空或只有一个元素
}
~CircularLinkedList() { // 析构函数释放内存
Node* current = head;
while (current) {
Node* temp = current;
current = current->next;
delete temp;
}
head = nullptr;
}
};
int main() {
CircularLinkedList list;
list.addNode(1);
list.addNode(2);
list.addNode(3);
list.display(); // 输出: 1 2 3
list.removeNode(2);
list.display(); // 输出: 1 3
return 0;
}
```
阅读全文
相关推荐
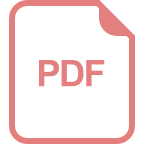
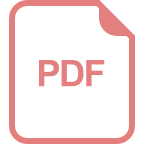
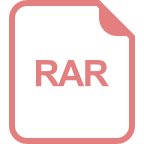
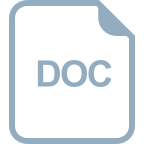
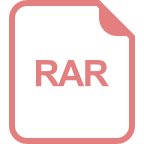
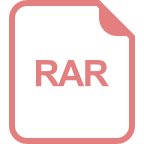
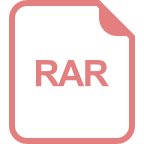
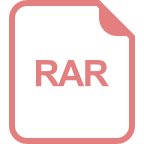
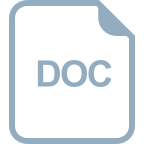
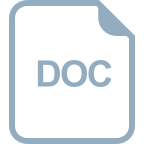
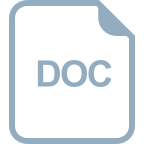
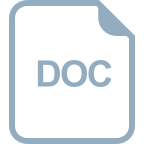
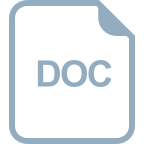
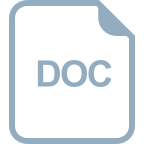
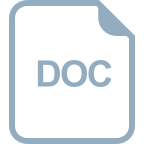




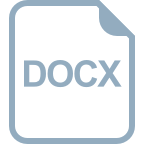