约瑟夫问题C++单向循环链表分文件数据结构
时间: 2023-08-04 16:34:14 浏览: 117
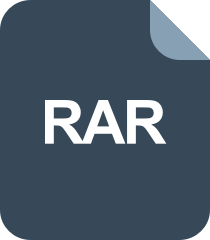
用C++做的单循环链表简单表示约瑟夫问题
好的,我可以帮你解答这个问题。
首先,让我们来了解一下约瑟夫问题的背景。约瑟夫问题是一个经典的问题,据传是由古代历史学家约瑟夫斯(Josephus)提出的。问题的具体描述如下:
有n个人围成一圈,从第一个人开始报数,报到m的人出圈,剩下的人继续从1开始报数,直到剩下最后一个人。求出最后一个出圈的人的编号。
为了解决这个问题,我们可以使用单向循环链表来实现。下面是一个简单的C++单向循环链表的实现,包含了三个文件:Node.h、List.h和List.cpp。
首先是Node.h文件,用来定义节点类:
```cpp
// Node.h
#ifndef NODE_H
#define NODE_H
class Node {
public:
int data;
Node* next;
Node(int data) {
this->data = data;
next = nullptr;
}
};
#endif
```
然后是List.h文件,用来定义链表类:
```cpp
// List.h
#ifndef LIST_H
#define LIST_H
#include "Node.h"
class List {
public:
List();
~List();
void insert(int data);
void remove(Node* node);
Node* getHead();
Node* getNext(Node* node);
private:
Node* head;
};
#endif
```
最后是List.cpp文件,用来实现链表类的方法:
```cpp
// List.cpp
#include "List.h"
List::List() {
head = nullptr;
}
List::~List() {
Node* curr = head;
while (curr != nullptr) {
Node* temp = curr;
curr = curr->next;
delete temp;
}
}
void List::insert(int data) {
Node* node = new Node(data);
if (head == nullptr) {
head = node;
head->next = head;
}
else {
Node* curr = head;
while (curr->next != head) {
curr = curr->next;
}
curr->next = node;
node->next = head;
}
}
void List::remove(Node* node) {
Node* curr = head;
if (node == head) {
head = head->next;
}
else {
while (curr->next != node) {
curr = curr->next;
}
curr->next = node->next;
}
delete node;
}
Node* List::getHead() {
return head;
}
Node* List::getNext(Node* node) {
return node->next;
}
```
现在我们就可以使用这个单向循环链表来解决约瑟夫问题了。下面是一个简单的解决方案:
```cpp
#include <iostream>
#include "List.h"
int josephus(int n, int m) {
List list;
for (int i = 1; i <= n; i++) {
list.insert(i);
}
Node* curr = list.getHead();
while (n > 1) {
for (int i = 1; i < m; i++) {
curr = list.getNext(curr);
}
Node* temp = curr;
curr = list.getNext(curr);
list.remove(temp);
n--;
}
return list.getHead()->data;
}
int main() {
int n = 5;
int m = 3;
int result = josephus(n, m);
std::cout << "The last person is " << result << std::endl;
return 0;
}
```
这个程序将输出:
```
The last person is 4
```
这就是使用C++单向循环链表解决约瑟夫问题的一个简单的例子。希望能对你有所帮助!
阅读全文
相关推荐
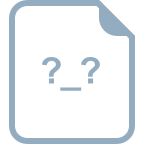

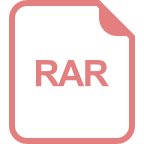
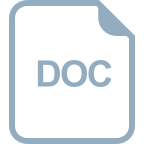
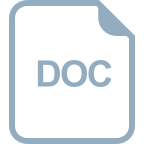
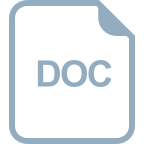
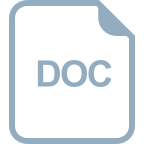
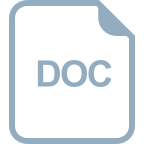
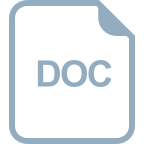
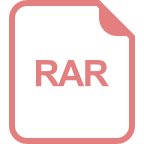
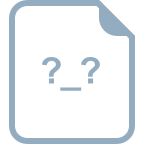
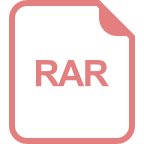
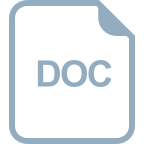
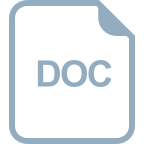
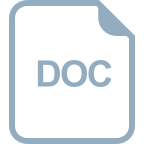
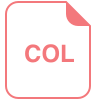
