如何使用Selenium库有效地抓取和模拟填写网页上的动态验证码输入框?
时间: 2024-11-13 21:24:06 浏览: 23
使用Selenium库抓取和模拟动态验证码输入框通常需要结合一些额外的技巧,因为验证码通常是设计来防止自动化工具访问的。以下是基本步骤:
1. **安装Selenium**:
首先,你需要确保已经安装了Python的selenium库以及对应的浏览器驱动(如ChromeDriver、FirefoxDriver等)。可以使用pip安装:
```
pip install selenium
```
2. **初始化浏览器和会话**:
```python
from selenium import webdriver
driver = webdriver.Chrome() # 或者替换为你选择的浏览器
driver.get('目标网页URL')
```
3. **定位动态元素**:
动态验证码通常不会出现在HTML源码中,而是通过JavaScript生成。你可以使用`WebDriverWait`和`ExpectedConditions`等待条件来获取元素:
```python
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
captcha_input = WebDriverWait(driver, 10).until(
EC.presence_of_element_located((By.XPATH, '//*[@id="dynamic_captcha"]'))
)
```
4. **处理验证码**:
如果验证码是一个图像,可能需要用OCR技术识别文字;如果是滑动验证码或颜色匹配等其他类型的动态验证,可能需要用户手动操作。这里仅示例图像验证码识别:
```python
from pytesseract import image_to_string
captcha_image = captcha_input.screenshot_as_png
captcha_text = image_to_string(captcha_image)
```
5. **填充验证码**:
将识别出的文字填入到输入框内:
```python
captcha_input.send_keys(captcha_text)
```
6. **提交表单**:
完成验证码输入后,提交表单:
```python
submit_button = driver.find_element(By.ID, "submit")
submit_button.click()
```
7. **异常处理**:
考虑加入适当的异常处理,如网络错误、验证码无法识别等情况。
阅读全文
相关推荐
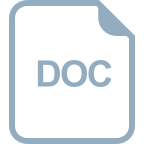
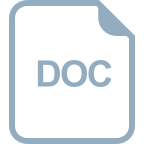
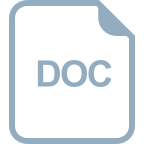
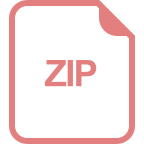
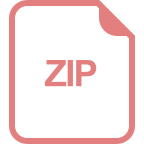
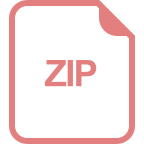
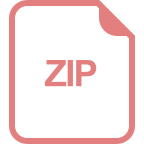
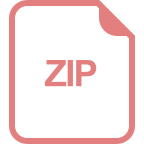
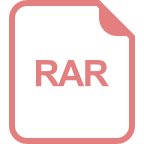
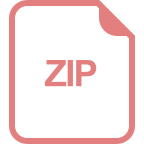
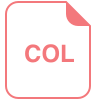
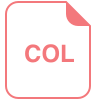
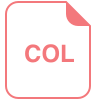
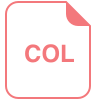
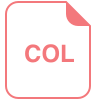
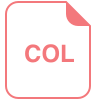
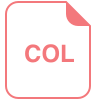

