使用Selenium进行模拟登录
发布时间: 2024-01-31 03:25:55 阅读量: 78 订阅数: 22 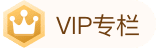
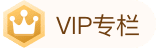
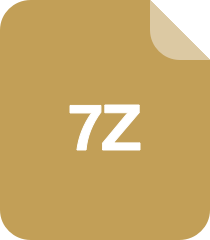
C# 使用Selenium模拟浏览器获取CSDN博客内容
# 1. 引言
## 模拟登录的概念
在网络应用中,登录是用户访问私有或受限制内容的一种常见方式。用户需要提供有效的凭据(如用户名和密码)来验证身份,以便使用特定的功能或获取特定的信息。而模拟登录则是一种通过编程的方式自动完成登录流程的方法,可以节省人工操作的时间和精力。
## Selenium的介绍
Selenium是一个广泛应用于Web应用自动化测试的工具,也可以用于模拟登录。它支持多种浏览器,如Chrome、Firefox、Safari等,并且提供了丰富的API,使得我们可以通过代码控制浏览器的行为。
通过使用Selenium,我们可以实现自动打开浏览器、输入用户名和密码、点击登录按钮等操作,从而实现模拟登录的效果。无论是测试网站的登录功能还是自动化爬取需要登录才能访问的信息,Selenium都是一个强大而实用的工具。
下面,我们将介绍如何进行模拟登录的准备工作。
# 2. 准备工作
在开始使用Selenium进行模拟登录之前,我们需要进行一些必要的准备工作。
### 环境搭建
首先,我们需要确保我们的机器上已经安装了Python解释器。Selenium是一个基于Python开发的自动化测试工具,所以我们需要先确保Python环境正常运行。
其次,我们需要安装浏览器驱动。Selenium需要与特定的浏览器进行交互,所以我们需要下载相应的浏览器驱动并进行配置。不同的浏览器有不同的驱动,我们可以通过官方文档来查找并下载对应的驱动。
### 安装Selenium库
Selenium库是用于操作浏览器的一个Python库,我们需要通过安装来获取该库。
对于Python的安装包管理工具pip,我们可以直接使用以下命令来安装Selenium库:
```bash
pip install selenium
```
### 配置浏览器驱动
安装了浏览器驱动之后,我们需要将驱动文件所在的路径添加到系统的环境变量中,以便让Selenium能够找到对应的驱动。
在Windows系统中,我们可以通过以下步骤来配置环境变量:
1. 在桌面上右键点击"此电脑",选择"属性"。
2. 在弹出的窗口中,选择"高级系统设置"。
3. 在"系统属性"窗口中,点击"环境变量"按钮。
4. 在"系统变量"窗口中,找到名为"Path"的变量,双击进行编辑。
5. 在"编辑环境变量"窗口中,点击"新建"按钮,将驱动文件所在的路径添加进去。
6. 点击"确定"保存修改。
在Linux或MacOS系统中,我们可以编辑`~/.bash_profile`或`~/.bash_rc`文件,将驱动文件所在的路径添加到`PATH`环境变量中。然后运行以下命令使配置生效:
```bash
source ~/.bash_profile
```
至此,我们已经完成了Selenium的准备工作。在下一章节中,我们将会介绍如何使用Selenium进行元素定位。
# 3. 定位元素
在使用Selenium模拟登录之前,我们首先需要了解如何定位网页中的元素。元素定位是Selenium中非常重要的一环,它决定了我们能否准确地找到需要操作的元素。
#### 3.1 Selenium中的元素定位方法
Selenium提供多种元素定位的方法,我们可以根据具体的需求选择适当的方法。以下是Selenium中常用的元素定位方法:
- **ID定位**:使用元素的ID属性进行定位,通常来说ID是唯一的。
- **XPath定位**:使用元素在HTML文档中的路径进行定位,XPath可以通过元素标签、属性、相对位置等多种方式进行定位。
- **CSS选择器**:使用元素的CSS属性进行定位。
- **类名定位**:根据元素的class属性进行定位,通常会有多个元素具有相同的类名。
- **链接文本定位**:根据链接的文本信息进行定位,适用于需要点击链接的场景。
- **标签名称定位**:根据元素的标签名称进行定位,适用于查找某一类型的元素。
- **部分链接文本定位**:根据链接文本的部分信息进行定位,与链接文本定位类似,但不需要完全匹配。
下面我们重点介绍两种常用的元素定位方式:CSS选择器和XPath定位。
#### 3.2 CSS选择器
CSS选择器是一种通过元素的CSS属性来定位的方法,它使用一些特定的符号来表示选择器的规则。在Selenium中,可以使用`find_element_by_css_selector()`方法来使用CSS选择器定位元素。
假设我们需要定位登录页面的用户名输入框,可以使用以下的CSS选择器:
```python
driver.find_element_by_css_selector("#username")
```
在上述代码中,`#username`表示通过ID属性为"username"的元素进行定位。
除了ID选择器,CSS选择器还可以使用其他属性、标签名以及组合选择器等方式进行定位。例如,我们可以使用以下的CSS选择器来定位登录页面的登录按钮:
```python
driver.find_element_by_css_selector("button.login-btn")
```
在上述代码中,`button.login-btn`表示查找标签名为"button"且类名为"login-btn"的元素。
#### 3.3 XPath定位
XPath是一种通过元素的路径来定位的方法,它使用一些特定的语法规则来表示选择器的路径。在Selenium中,可以使用`find_element_by_xpath()`方法来使用XPath定位元素。
假设我们需要定位登录页面的密码输入框,可以使用以下的XPath定位:
```python
driver.find_element_by_xpath("//input[@name='password']")
```
在上述代码中,`//input[@name='password']`表示查找`name`属性为"password"的`input`元素。
XPath定位还可以使用元素的标签名、CSS选择器的属性、父子关系、兄弟关系等多种方式进行定位。例如,我们可以使用以下的XPath定位来定位登录页面的登录按钮:
```python
driver.find_element_by_xpath("//button[contains(@class, 'login-btn')]")
```
在上述代码中,`//button[contains(@class, 'login-btn')]`表示查找类名包含"login-btn"的`but
0
0
相关推荐







